To create a digital countdown timer with a graphical display, follow these steps:
- Create a new Unity 2D project.
- Import the CountdownTimer script and the red_square and green_square images into this project.
- Add a UI Text GameObject to the scene with a Font size of 30 and placeholder text such as a UI Slider value (this text will be replaced with the slider value when the scene starts). Set Horizontal- and Vertical- Overflow to Overflow.
- In the Hierarchy window, add a Slider GameObject to the scene by going to GameObject | UI | Slider.
- In the Inspector window, modify the settings for the position of the Slider GameObject's Rect Transform to the top-middle part of the screen.
- Ensure that the Slider GameObject is selected in the Hierarchy window.
- Deactivate the Handle Slide Area child GameObject (by unchecking it).
- You'll see the "drag circle" disappear in the Game window (the user will not be dragging the slider since we want this slider to be display-only):
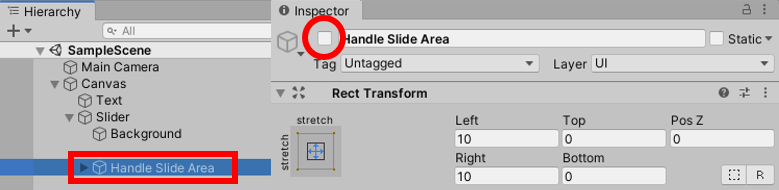
Figure 2.19 – Ensuring Handle Slide Area is deactivated
- Select the Background child and do the following:
- Drag the red_square image into the Source Image property of the Image (Script) component in the Inspector window.
- Select the Fill child of the Fill Area child and do the following:
- Drag the green_square image into the Source Image property of the Image (Script) component in the Inspector window.
- Select the Fill Area child and do the following:
- In the Rect Transform component, use the Anchors preset position of left-middle.
- Set Width to 155 and Height to 12:
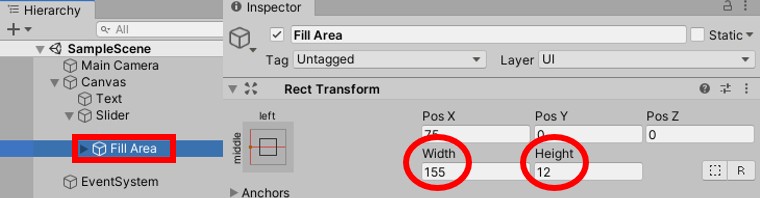
Figure 2.20 – Selections in the Rect Transform component
- Create a C# script class called SliderTimerDisplay that contains the following code and add an instance as a scripted component to the Slider GameObject:
using UnityEngine; using UnityEngine.UI; [RequireComponent(typeof(CountdownTimer))] public class SliderTimerDisplay : MonoBehaviour { private CountdownTimer countdownTimer; private Slider sliderUI; void Awake() { countdownTimer = GetComponent<CountdownTimer>(); sliderUI = GetComponent<Slider>(); } void Start() { SetupSlider(); countdownTimer.ResetTimer( 30 ); } void Update () { sliderUI.value = countdownTimer.GetProportionTimeRemaining(); print (countdownTimer.GetProportionTimeRemaining()); } private void SetupSlider () { sliderUI.minValue = 0; sliderUI.maxValue = 1; sliderUI.wholeNumbers = false; } }
Run your game. You will see the slider move with each second, revealing more and more of the red background to indicate the time remaining.