-
Book Overview & Buying
-
Table Of Contents
-
Feedback & Rating
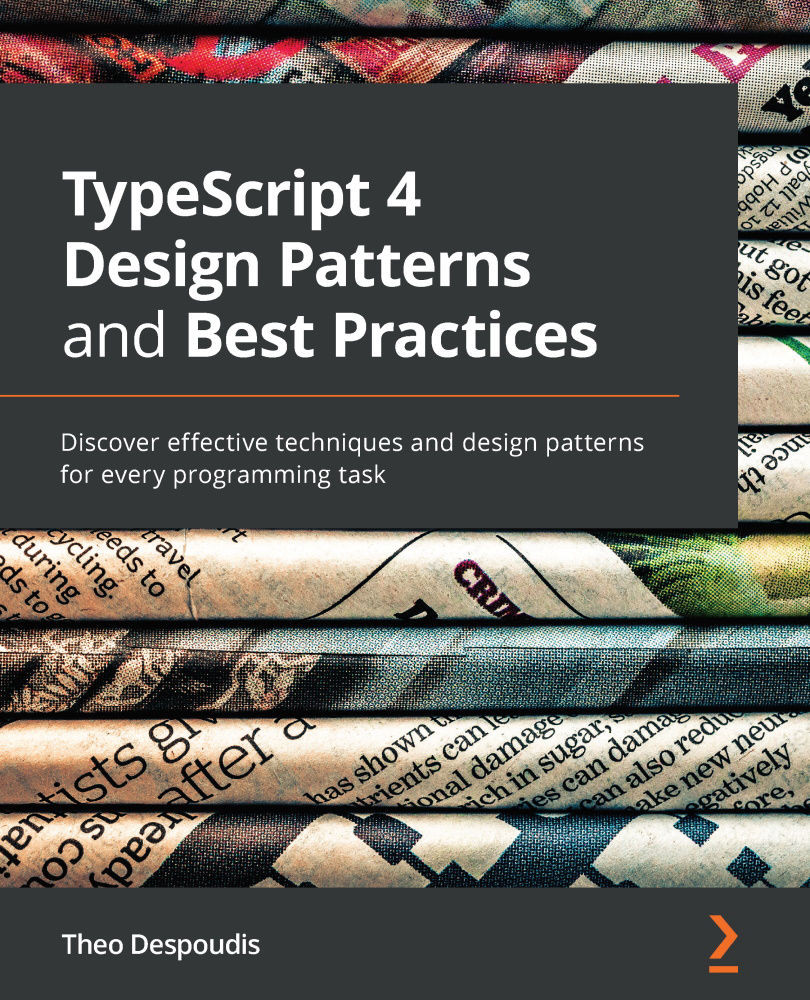
TypeScript 4 Design Patterns and Best Practices
By :
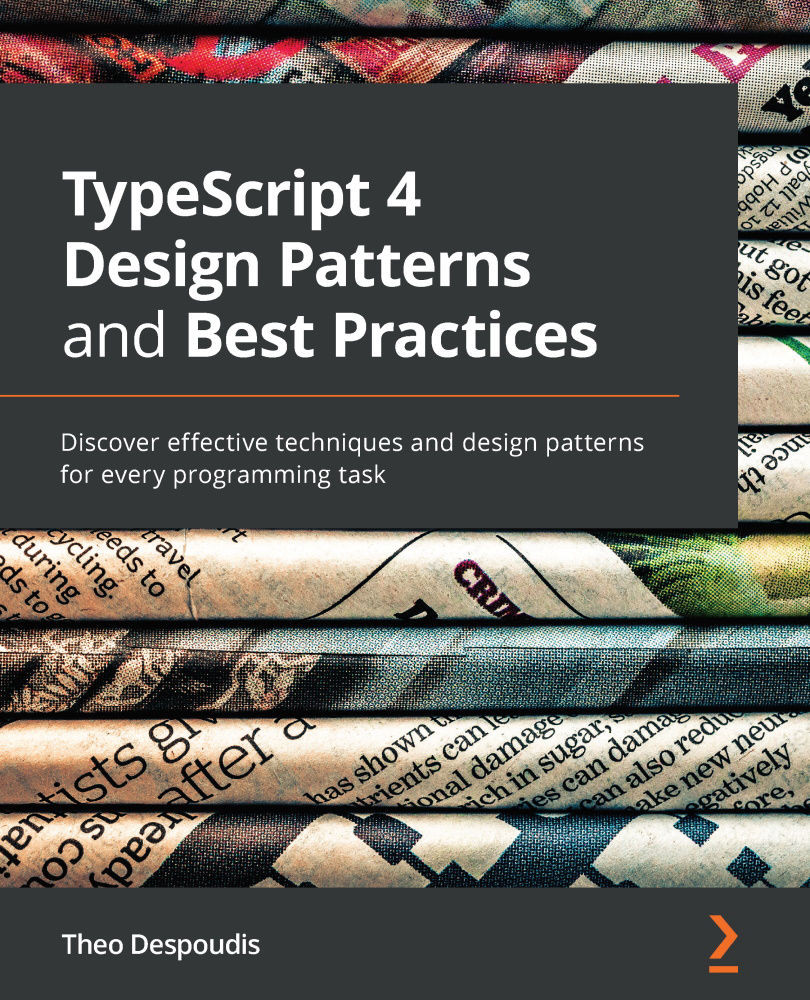
Transducers are another name for reducers that take an input and return another reducer, forming a composition between them. To understand why this is helpful, we'll explain the basics of reducers first.
A reducer is a simple function that accepts an input of type T
, which is typically a collection. It is a function that accepts the current value in the collection, the current aggregated value, and a starting value. The job of the reducer is to iterate over the collection starting from the initial value, calling the function that accepts the current aggregate and the current iteration and returns the end result.
Here is an example reducer in TypeScript:
Transducer.ts
const collection = [1, 2, 3, 4, 5]; function addReducer(curr: number, acc: number): number { return curr + acc; } console.log(collection.reduce(addReducer, 0));
This reducer function has the type (number, number): number
and the reduce
method accepts the reducer...