-
Book Overview & Buying
-
Table Of Contents
-
Feedback & Rating
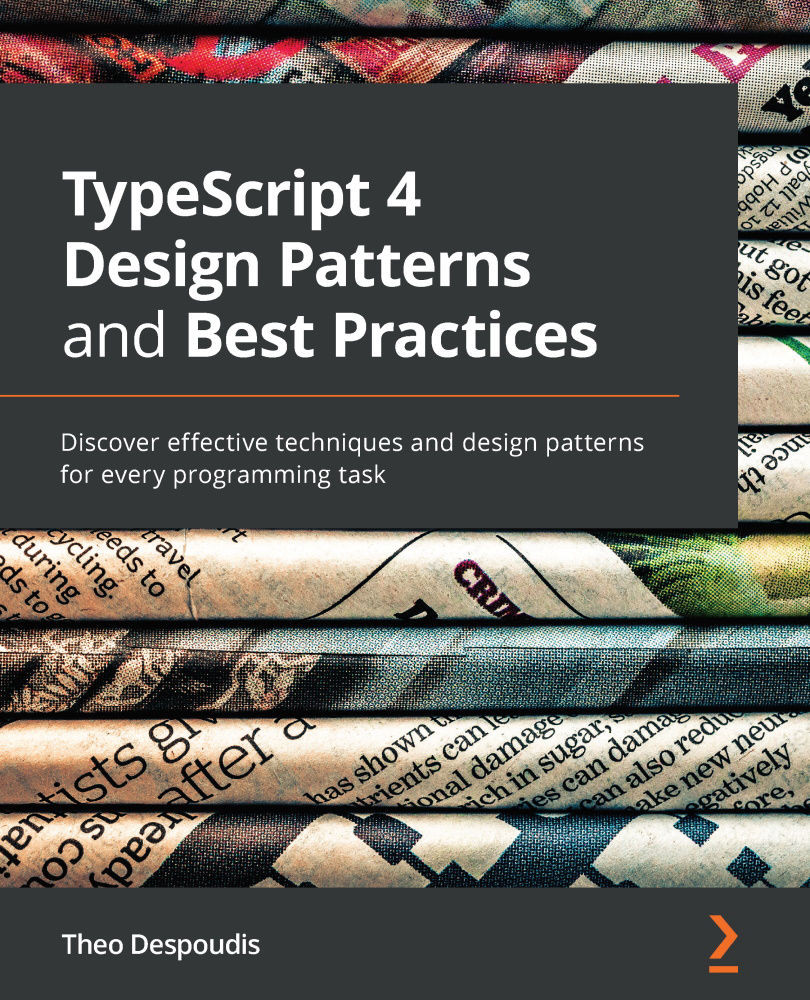
TypeScript 4 Design Patterns and Best Practices
By :
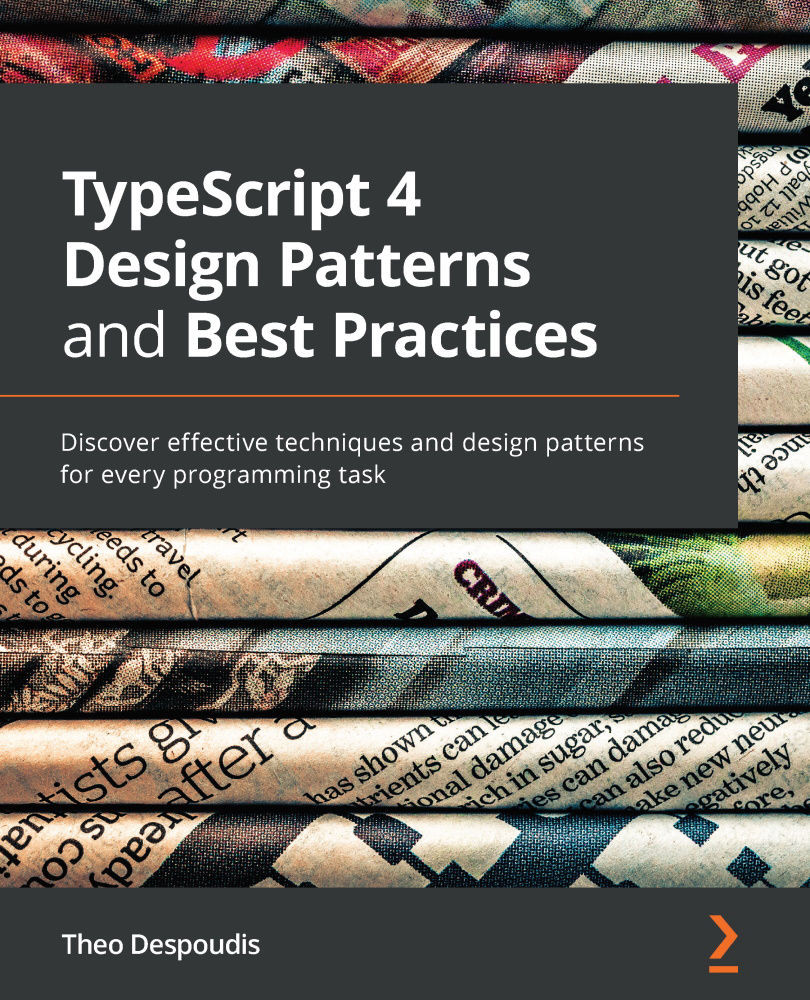
When working with type inference in TypeScript, sometimes you need to be explicit in regard to types, but most of the time, implicit typing is preferred. There are some caveats that you need to be aware of. We'll explain when it makes sense to use inference.
In TypeScript, you can either declare types for variables or instances explicitly or implicitly. Here is an example of explicit typing:
const arr: number[] = [1,2,3]
On the other hand, implicit typing is when you don't declare the type of the variable and let the compiler infer it:
const arr = [1,2,3]// type of arr inferred as number[]
If you declare and do not assign any value within the same line, then TypeScript will infer it as any
:
let x; // fails with noImplicitAny flag enabled x = 2;
This will fail to compile with the noImplicitAny
flag, so in that case, it's recommended to always declare the expected type of the variable.
Another case is when you declare a function...