-
Book Overview & Buying
-
Table Of Contents
-
Feedback & Rating
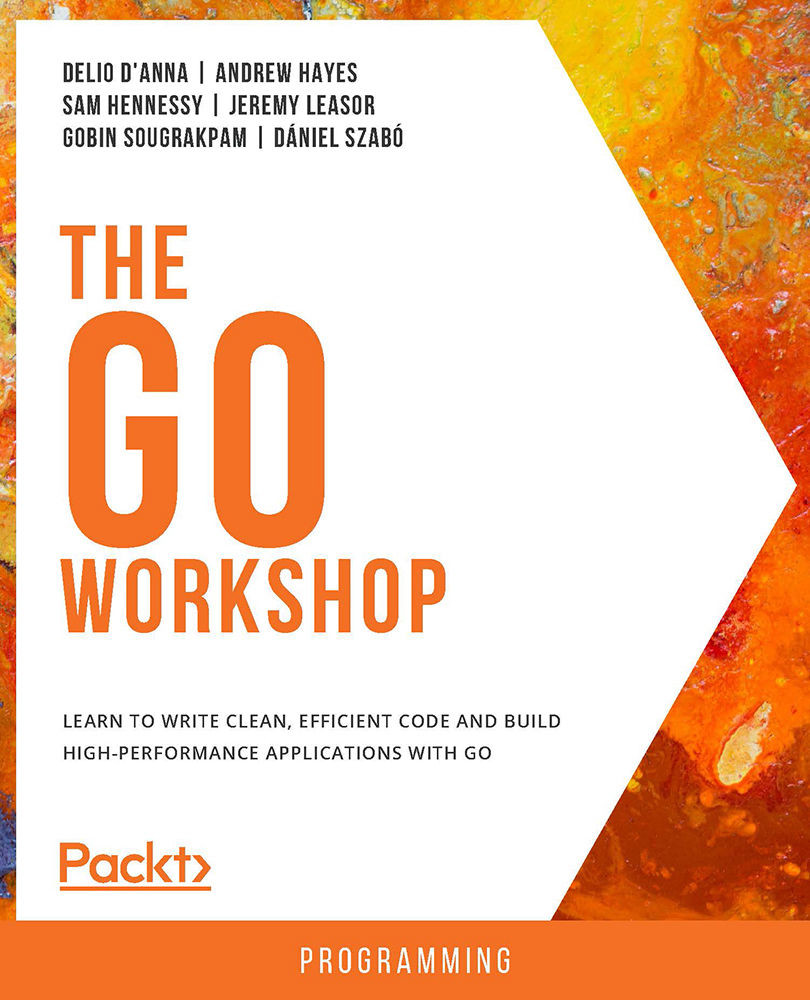
The Go Workshop
By :
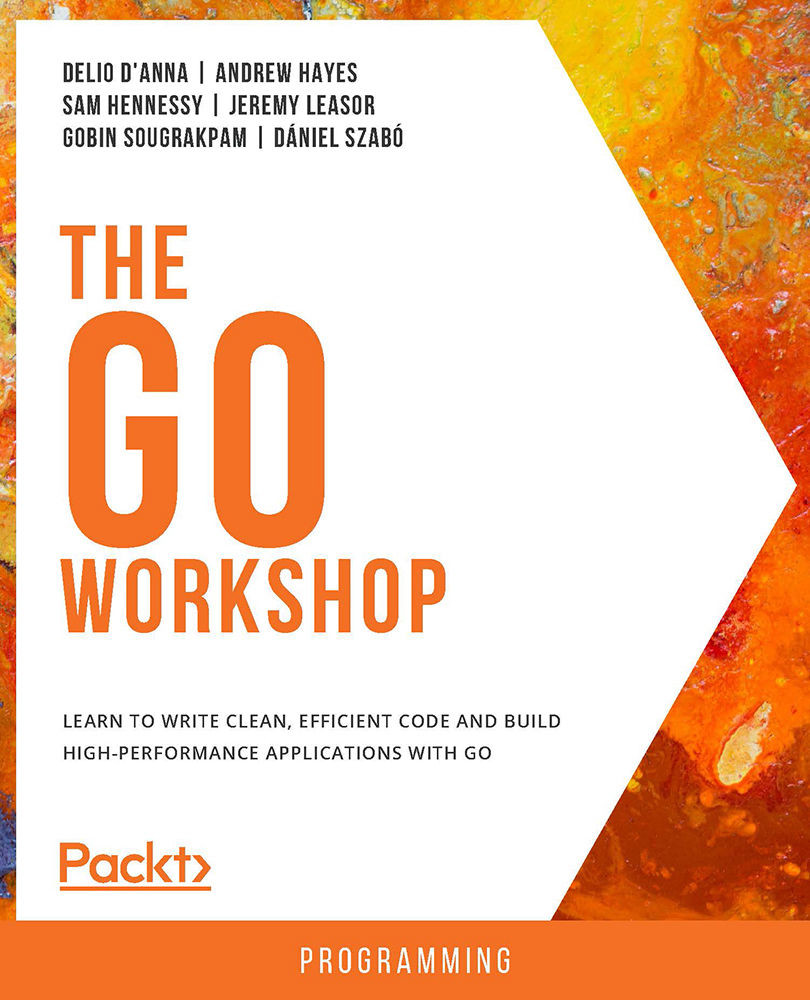
The Go Workshop
By:
Overview of this book
The Go Workshop will take the pain out of learning the Go programming language (also known as Golang). It is designed to teach you to be productive in building real-world software. Presented in an engaging, hands-on way, this book focuses on the features of Go that are used by professionals in their everyday work.
Each concept is broken down, clearly explained, and followed up with activities to test your knowledge and build your practical skills.
Your first steps will involve mastering Go syntax, working with variables and operators, and using core and complex types to hold data. Moving ahead, you will build your understanding of programming logic and implement Go algorithms to construct useful functions.
As you progress, you'll discover how to handle errors, debug code to troubleshoot your applications, and implement polymorphism using interfaces. The later chapters will then teach you how to manage files, connect to a database, work with HTTP servers and REST APIs, and make use of concurrent programming.
Throughout this Workshop, you'll work on a series of mini projects, including a shopping cart, a loan calculator, a working hours tracker, a web page counter, a code checker, and a user authentication system.
By the end of this book, you'll have the knowledge and confidence to tackle your own ambitious projects with Go.
Table of Contents (21 chapters)
Preface
2. Logic and Loops
3. Core Types
4. Complex Types
5. Functions
6. Errors
7. Interfaces
8. Packages
9. Basic Debugging
10. About Time
11. Encoding and Decoding (JSON)
12. Files and Systems
13. SQL and Databases
14. Using the Go HTTP Client
15. HTTP Servers
16. Concurrent Work
17. Using Go Tools
18. Security
19. Special Features
Appendix
Customer Reviews