Variables are references to Python objects. They are created by assignments, for example:
a = 1
diameter = 3.
height = 5.
cylinder = [diameter, height] # reference to a list
Variables take names that consist of any combination of capital and small letters, the underscore _, and digits. A variable name must not start with a digit. Note that variable names are case sensitive. A good naming of variables is an essential part of documenting your work, so we recommend that you use descriptive variable names.
Python has 33 reserved keywords, which cannot be used as variable names (see Table 2.1). Any attempt to use such a keyword as a variable name would raise a syntax error:
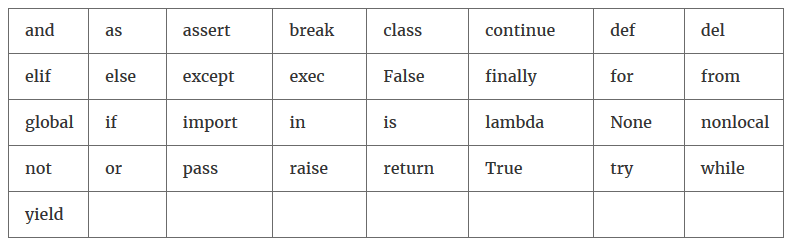
Table 2.1: Reserved Python keywords
As opposed to other programming languages, variables require no type declaration in Python. The type is automatically deduced:
x = 3 # integer (int)
y = 'sunny' # string (str)
You can create several variables with a multiple assignment statement:
a = b = c = 1 # a, b and c get the same value 1
Variables can also be altered after their definition:
a = 1
a = a + 1 # a gets the value 2
a = 3 * a # a gets the value 6
The last two statements can be written by combining the two operations with an assignment directly by using increment operators:
a += 1 # same as a = a + 1
a *= 3 # same as a = 3 * a