-
Book Overview & Buying
-
Table Of Contents
-
Feedback & Rating
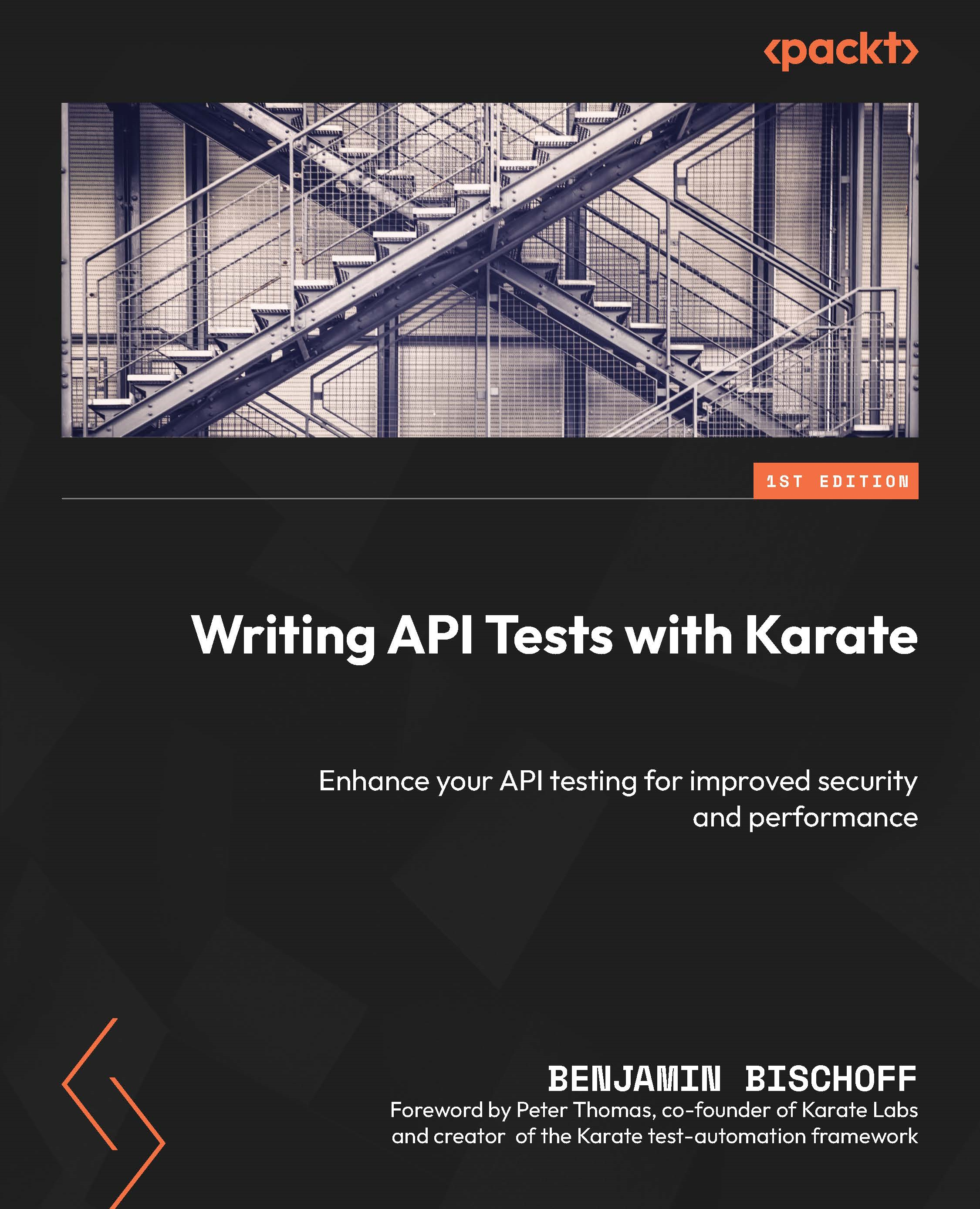
Writing API Tests with Karate
By :
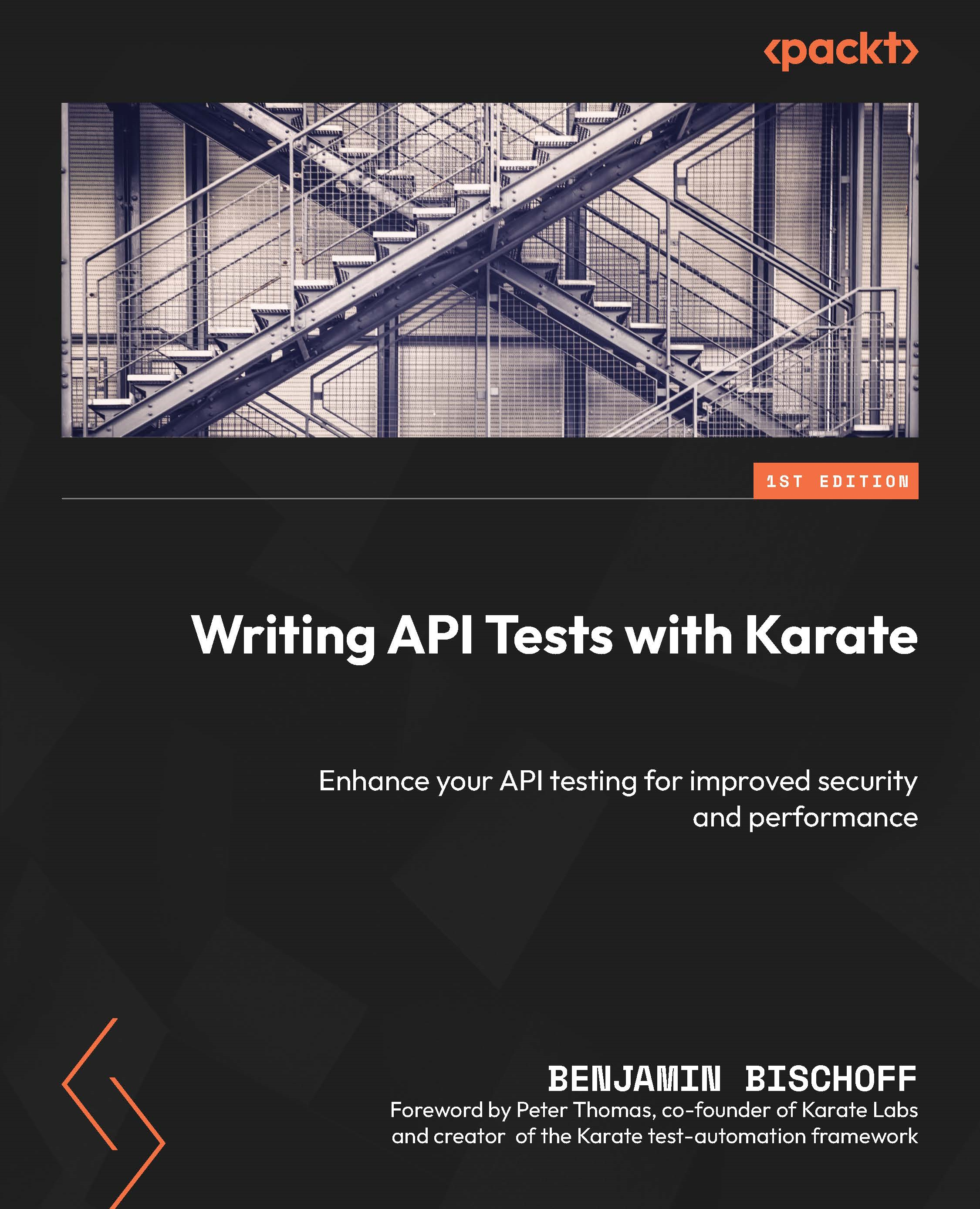
One of the strengths of the Karate framework is its native support for a variety of different data types that are typically associated with API payloads and responses. That means that it is possible to use them in their original form within test scripts without the need of escaping certain characters or converting them to be usable.
Let’s look at some widely used data formats here and the way they would represent data about this book and the first chapter for comparison.
JSON is pretty much the standard exchange format for REST APIs. Additionally, it is the standard format for GraphQL. It is small, structured, and human-readable while being a great format to work with JavaScript because it can be used natively there. This is a simple JSON example that shows how data can be represented by nesting keys and values:
{ "book": { "title": "Writing tests with Karate", "author": "Benjamin Bischoff", "chapters": [{ "number": "1", "title": "Karate's core concepts" }] } }
This is the format we will encounter most throughout the book, as Karate can also represent other formats through JSON and make them testable.
GraphQL was developed by Facebook and released to the public in 2015. In 2018, it was transferred to the newly formed GraphQL foundation under the Linux Foundation (https://www.linuxfoundation.org/).
GraphQL’s request and response format is JSON. Contrary to a REST API that always returns fixed data structures, users can define exactly what data they need in each request and only the requested data is then returned. This saves bandwidth and enables fetching deeply nested data without making multiple requests in a row. From a structural point of view, the only thing different from JSON responses is the added data
object that wraps the expected JSON response:
{ data: { "book": { "title": "Writing tests with Karate", "author": "Benjamin Bischoff", "chapters": [{ "number": "1", "title": "Karate's core concepts" }] } } }
In Chapter 6, More Advanced Karate Features, we will check out how to handle this within the Karate framework.
Like JSON, Extensible Markup Language (XML) is another human-readable format but with some more overhead for the same set of data. Karate handles this format equally well, though:
<?xml version="1.0" encoding="UTF-8" ?> <book> <title>Writing tests with Karate</title> <author>Benjamin Bischoff</author> <chapters> <chapter> <number>1</number> <title>Karate's core concepts</title> </chapter> </chapters> </book>
You can see that XML is more redundant than JSON because it uses similarly named opened and closed tags (e.g., <title>
and </title>
) to enclose values.
Yet another markup language (YAML) has a very minimalistic, indentation-based syntax. It is a rather new format that gained popularity mainly for its use in configuration files. The book example would look as follows, making this the most readable (but also error prone) format of them all:
--- book: title: Writing tests with Karate author: Benjamin Bischoff chapters: - number: 1 title: Karate's core concepts
Karate can work with the YAML format easily by automatically converting it into JSON!
Comma-separated values (CSV) files contain rows of data that are separated by line breaks. Each line is a dataset (or record) in which values are typically delimited by commas. It is often used as a data exchange format from and to spreadsheets, such as Excel:
title,author,chapter/number,chapter/title Writing tests with Karate,Benjamin Bischoff,1,Karate's core concepts
Like YAML, Karate converts CSV into JSON arrays automatically!
Luckily, Karate supports all textual formats. For those that are not included natively, we are free to use generic strings. However, depending on how obscure the format in question is, we need to write our own Java or JavaScript handling for such edge cases.
Through Karate’s Java interoperability, it is possible to handle virtually any binary file format. Additionally, Karate has the special bytes
type to convert any binary data into standard byte arrays to use as payloads.
If you need to use websocket
connections that use binary data, there is even a dedicated built-in method available, karate.webSocketBinary()
.
In this section, we have seen the different data formats that Karate can understand and work with. You can probably already see how powerful this framework is. Next, we will check how Karate’s JavaScript engine helps even further with this.