A macro called max is defined of size 100. Three arrays, p, q, and r, are defined of size max. You will first be asked to enter the size of the first array, p, followed by the sorted elements for array p. The process is repeated for the second array q.
Three indices, i, j and k, are defined and initialized to 0. The three indices will point to the elements of the three arrays, p, q, and r, respectively.
The first elements of arrays p and q, in other words, p[0] and q[0], are compared and the smaller one is assigned to array r.
Because q[0] is smaller than p[0], q[0] is added to array r, and the indices of arrays q and r are incremented for the next comparison as follows:
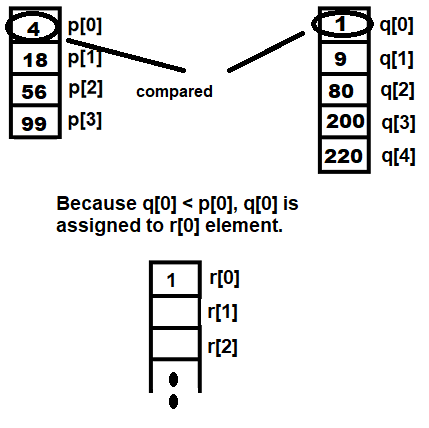
Figure 1.25
Next, p[0] will be compared with q[1]. Because p[0] is smaller than q[1], the value at p[0] will be assigned to array r at r[1]:
Figure 1.26
Then, p[1] will be compared with q[1]. Because q[1] is smaller than p[1], q[1] will be assigned to array r, and the indices of the q and r arrays will be incremented for the next comparisons (refer to the following diagram):
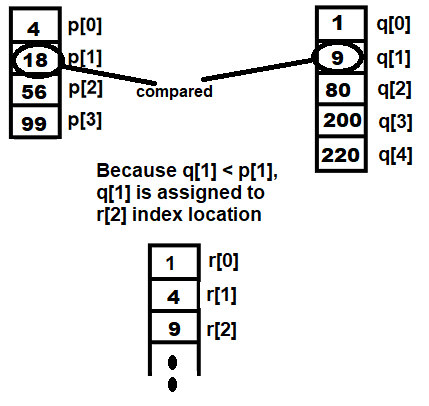
Figure 1.27
Let's use GCC to compile the mergetwosortedarrays.c program as follows:
D:\CBook>gcc mergetwosortedarrays.c -o mergetwosortedarrays
Now, let's run the generated executable file, mergetwosortedarrays.exe, in order to see the output of the program:
D:\CBook>./mergetwosortedarrays
Enter length of first array:4
Enter 4 elements of the first array in sorted order
4
18
56
99
Enter length of second array:5
Enter 5 elements of the second array in sorted order
1
9
80
200
220
The combined sorted array is:
1
4
9
18
56
80
99
200
220
Voilà! We've successfully merged two sorted arrays into one.