-
Book Overview & Buying
-
Table Of Contents
-
Feedback & Rating
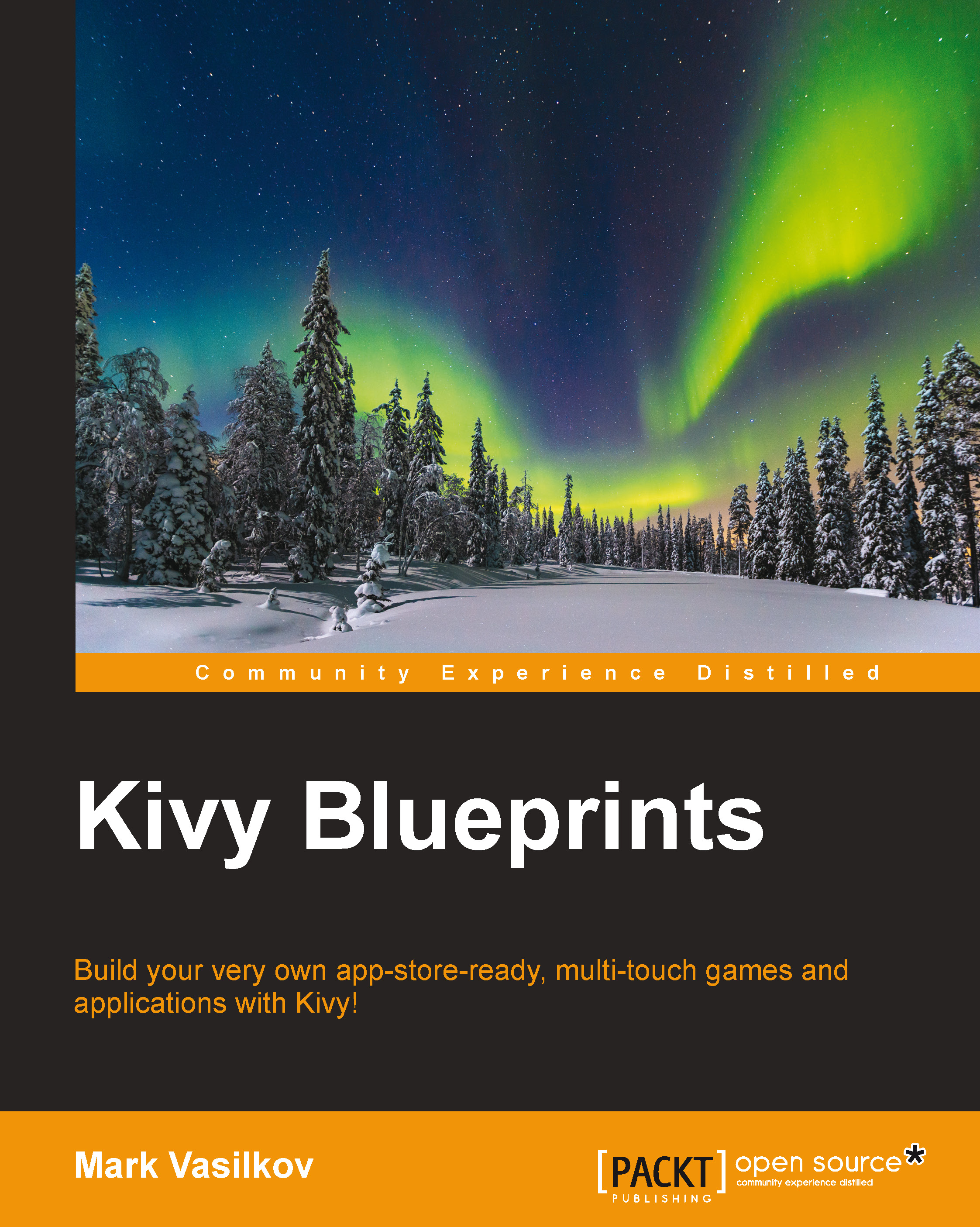
Kivy Blueprints
By :
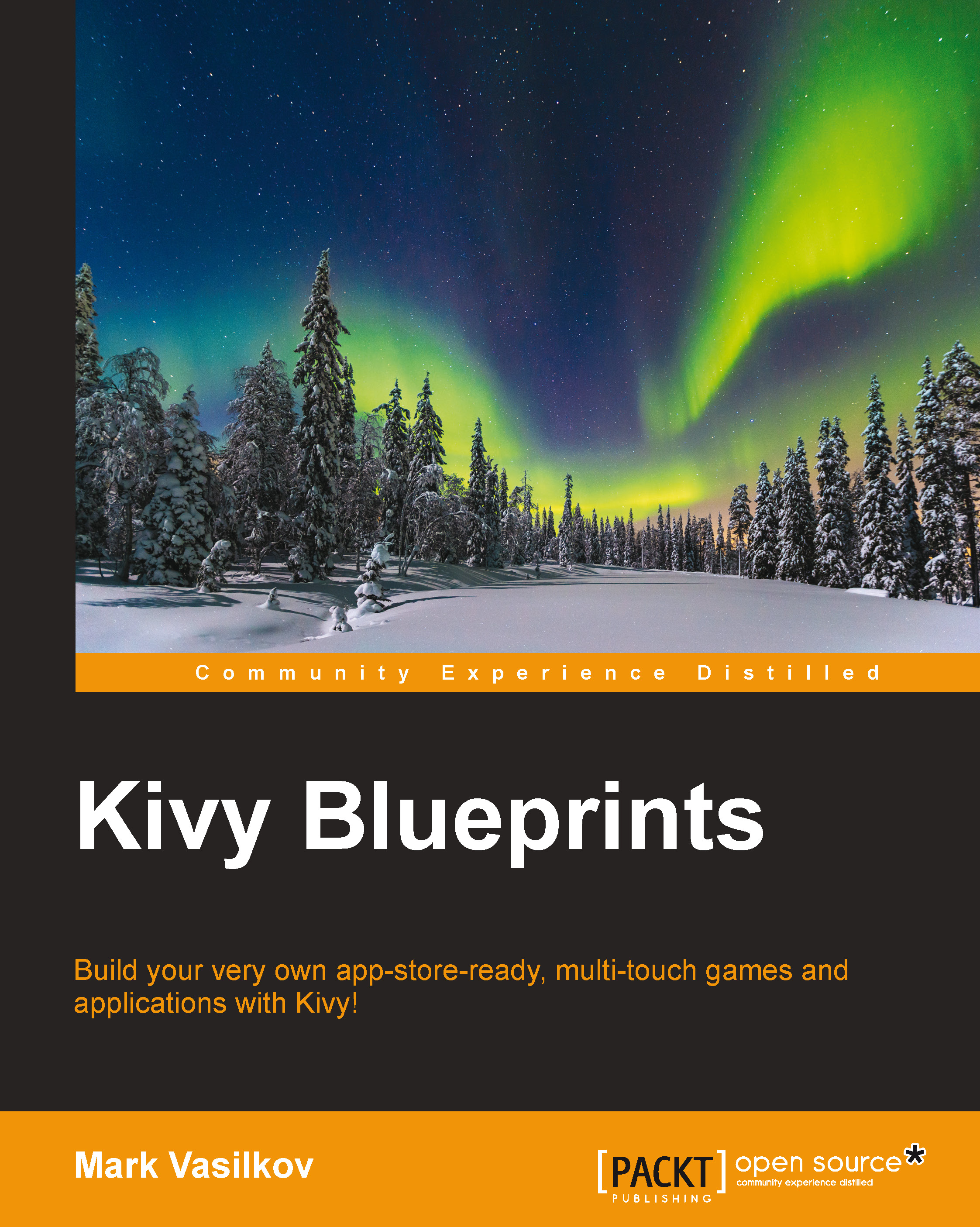
This book will walk you through the creation of nine little Kivy programs, each resembling a real-world use case for the Kivy framework. On many occasions, the framework will be utilized together with other Python modules fitting for the task at hand. We will see that Kivy provides a great deal of flexibility, allowing us to solve vastly different problems in a clean, concise manner.
Let's start small. In this chapter, we will build a simple Clock app, similar in concept to the built-in application found in both iOS and Android. In the first part of the chapter, we will create a non-interactive digital clock display and style it, giving our program an Android-ish flat look. We will also briefly discuss the event-driven program flow and a Kivy main loop, introducing timers used to perform recurring tasks, such as updating the screen every frame.
In the second part of this chapter, we will add a stopwatch display and controls, creating a fluid layout suitable for any screen size and orientation. A stopwatch, naturally, needs user interaction, which we are going to implement last.
The important topics introduced in this chapter are as follows:
Our finished program, depicted in the following screenshot, will only be about 60 lines long, split equally between a Python source code and a Kivy language (.kv
) interface definition file.
The final look of the Clock app we're going to build.