-
Book Overview & Buying
-
Table Of Contents
-
Feedback & Rating
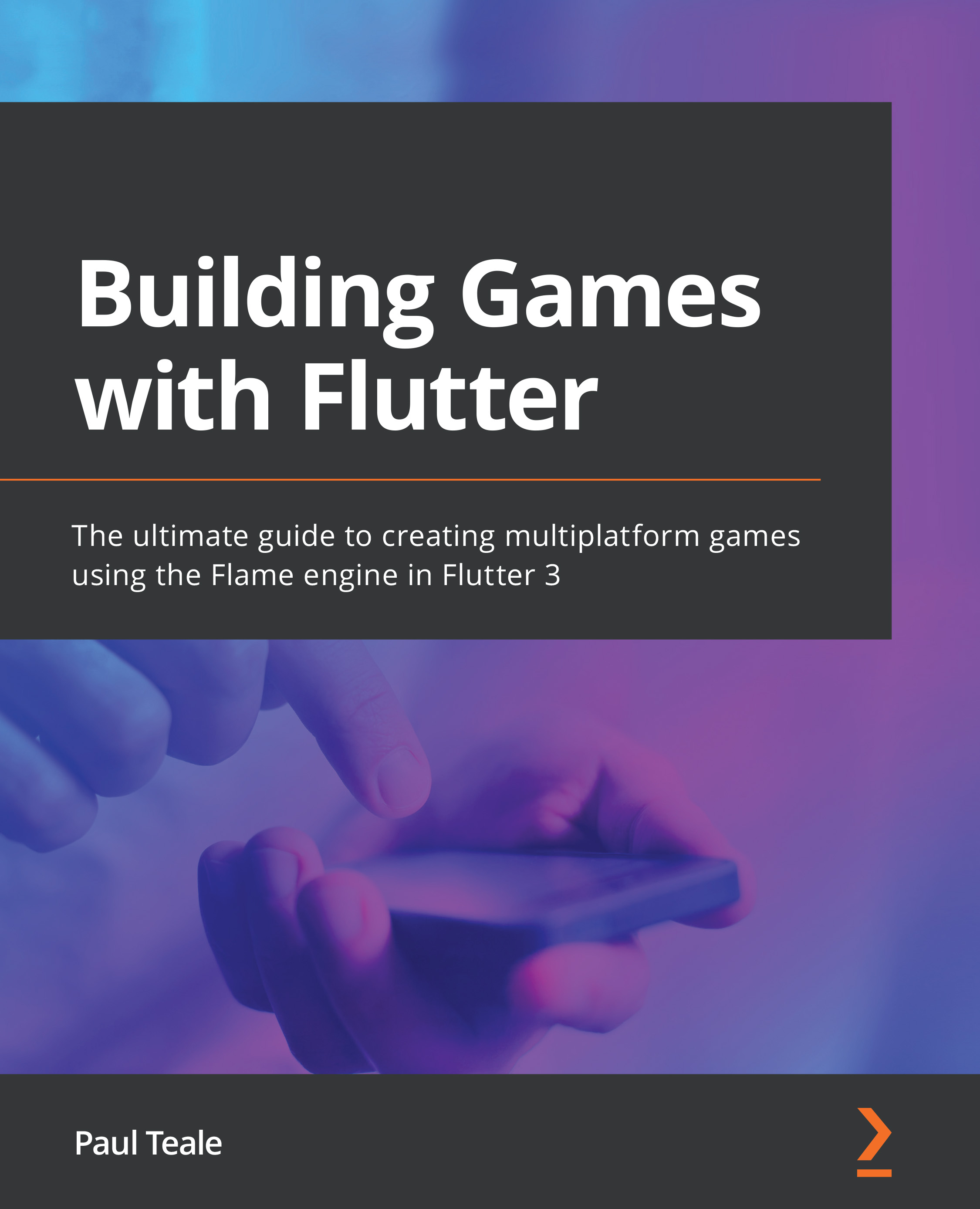
Building Games with Flutter
By :
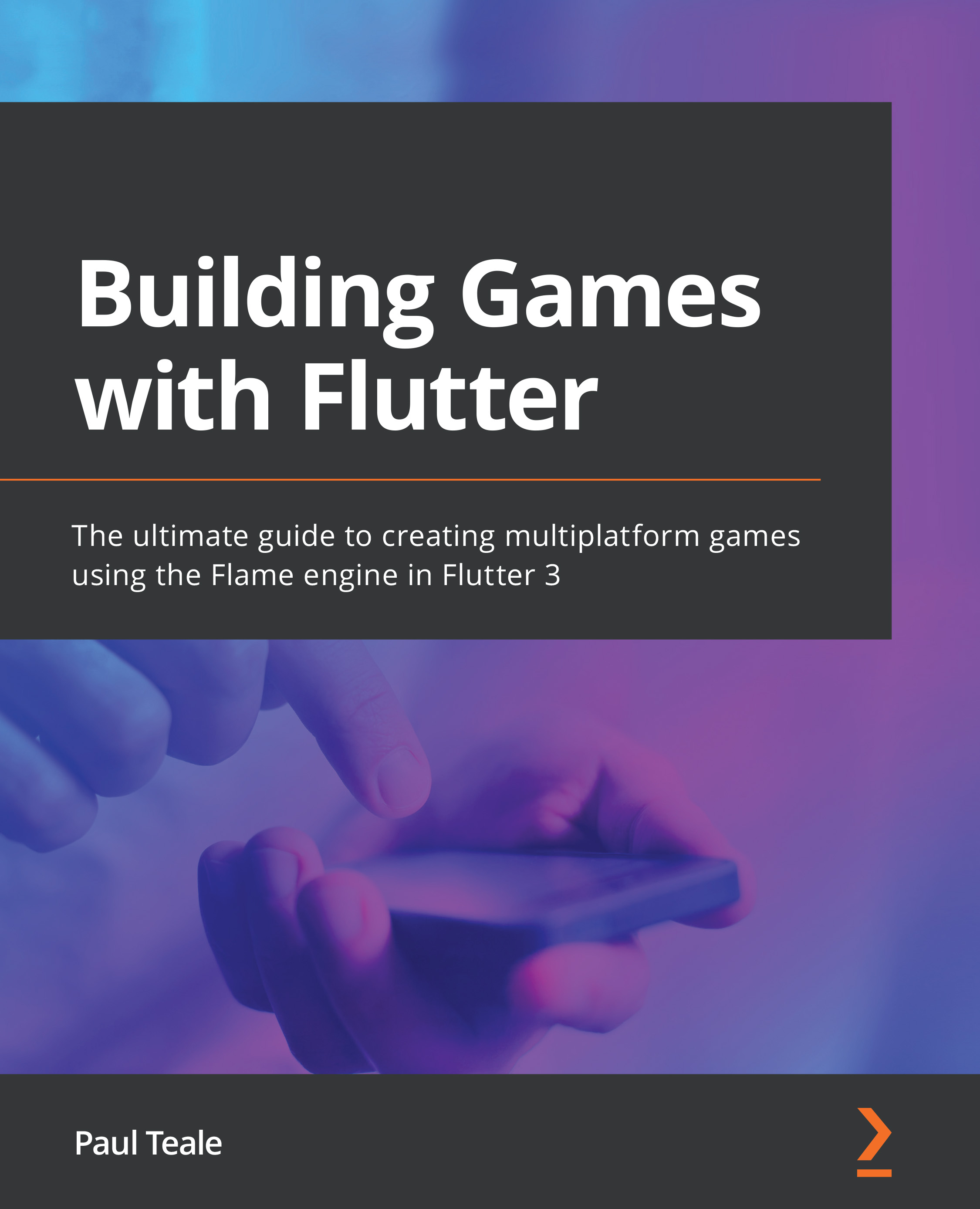
Here is a code sample for you to run to show how easy it is to draw and animate a simple shape.
To run this example, follow these steps:
flutter create goldrush
goldrush
folder that Flutter created in your code editor, and then open the pubspec.yaml
file.description: Flutter game from Building Games with Flutter
sdk: ">=2.17.0 <3.0.0"
This is the latest version of the SDK at the time of writing the book, and supports the latest features of Flutter and Dart.
Flame
(which we will talk more about in the next chapter):cupertino_icons: ^1.0.2 flame: 1.0.0
Flame is a great library and provides us with a lot of functionality needed to build games using Flutter and Dart.
pubspec.yaml
file, save the changes.flutter pub get
lib/main.dart
file and delete all the boilerplate code.import 'dart:ui'; import 'package:flame/flame.dart'; import 'package:flame/palette.dart'; import 'package:flutter/material.dart'; import 'package:flame/game.dart';
void main() async { final goldRush = GoldRush(); WidgetsFlutterBinding.ensureInitialized(); await Flame.device.fullScreen(); await Flame.device.setPortrait(); runApp( GameWidget(game: goldRush) ); }
Here, we set up our GoldRush
game object (which we will define next) and told Flame that we want to run the game in full screen and in portrait mode. We also ran the app, passing the GameWidget
.
class GoldRush with Loadable, Game { static const int squareSpeed = 250; static final squarePaint = BasicPalette.green.paint(); static final squareWidth = 100.0, squareHeight = 100.0; late Rect squarePos; int squareDirection = 1; late double screenWidth, screenHeight, centerX, centerY;
Let's break down what we did here:
250
; you can adjust this to a higher number to make the animation faster or lower to make the animation slower. 100
pixels.Rect
for the square position, which will be initialized in onLoad
once we have calculated the center of the screen for the starting position.x
value and move the box to the right.onLoad
function, we will calculate the center starting position of the box based on the screen size:@override Future<void> onLoad() async { super.onLoad(); screenWidth = MediaQueryData.fromWindow(window).size.width; screenHeight = MediaQueryData.fromWindow(window).size.height; centerX = (screenWidth / 2) - (squareWidth / 2); centerY = (screenHeight / 2) - (squareHeight / 2); squarePos = Rect.fromLTWH(centerX, centerY, squareWidth, squareHeight); }
@override void render(Canvas canvas) { canvas.drawRect(squarePos, squarePaint); }
Then, if the position of the square has reached the edge of the screen, we can flip the direction of the square:
@override void update(double deltaTime) { squarePos = squarePos.translate(squareSpeed * squareDirection * deltaTime, 0); if (squareDirection == 1 && squarePos.right > screenWidth) { squareDirection = -1; } else if (squareDirection == -1 && squarePos.left < 0) { squareDirection = 1; } } }
Now, we have gone through a simple animation example to show how easy it is to get started and to give you a feel for game programming with Flutter.
Feel free to play with the code, maybe changing the color of the square or adding more squares at a different position. In the next chapter, we will dig deeper into this code.