-
Book Overview & Buying
-
Table Of Contents
-
Feedback & Rating
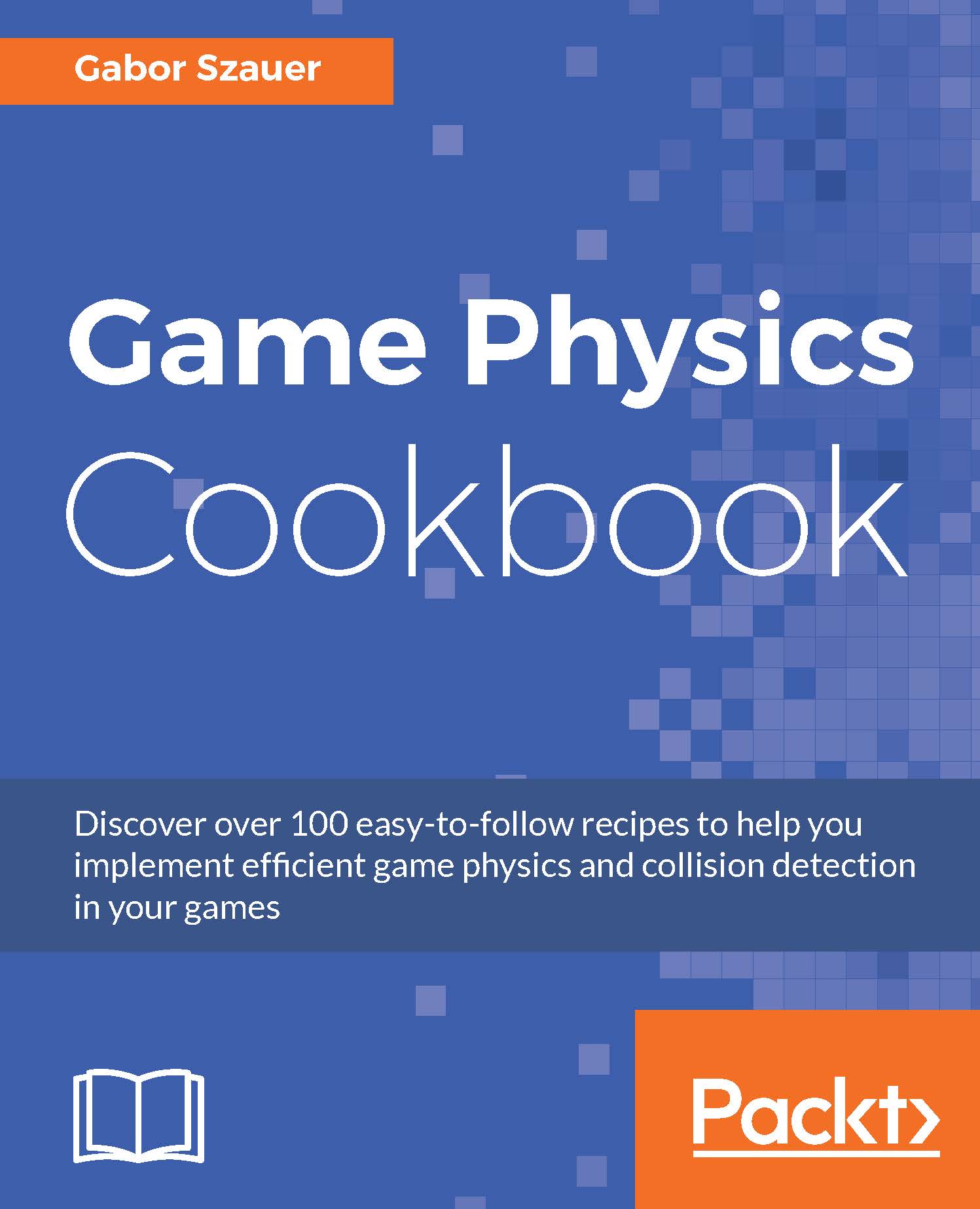
Game Physics Cookbook
By :
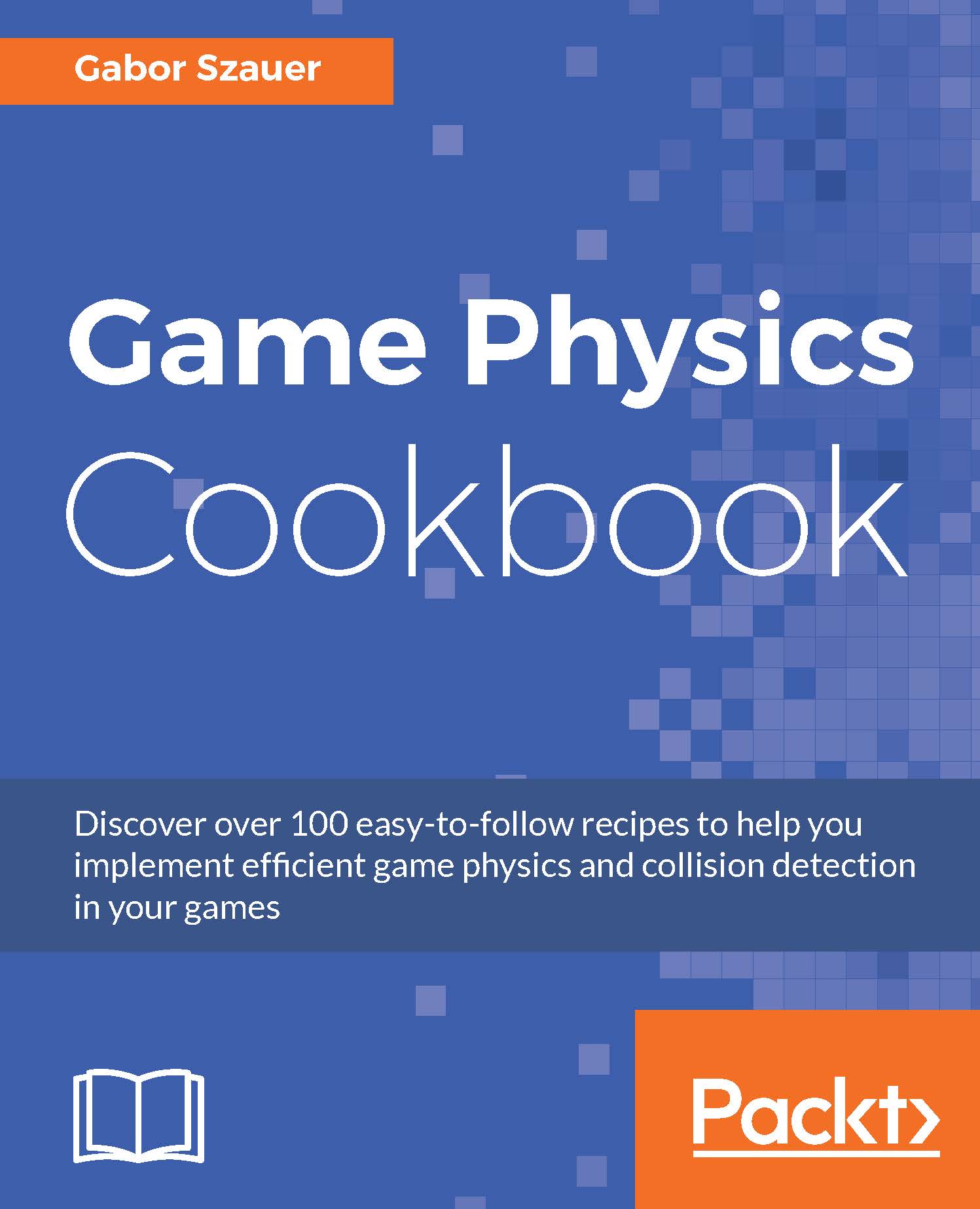
To check if a sphere intersects anything you follow a simple formula. Find the closest point to the sphere on the shape and use this point to find the distance between the sphere and the shape. Compare the resulting distance to the radius of the sphere. If the distance is less than the radius, there is a collision. Checking if a sphere and plane intersect follows this same formula:
We are going to implement a function to test if a sphere and a plane are intersecting. We will also use a #define
macro to implement convenience functions to see if a plane intersects a sphere. This macro just switches the function name and arguments around.
Follow the given steps to implement a sphere to plane intersection test:
Declare SpherePlane
in Geometry3D.h
:
bool SpherePlane(const Sphere& sphere, const Plane& plane);
Declare the PlaneSphere
macro in Geometry3D.h
:
#define PlaneSphere(plane, sphere) \ SpherePlane(sphere, plane)
Implement SpherePlane
in Geometry3D...