-
Book Overview & Buying
-
Table Of Contents
-
Feedback & Rating
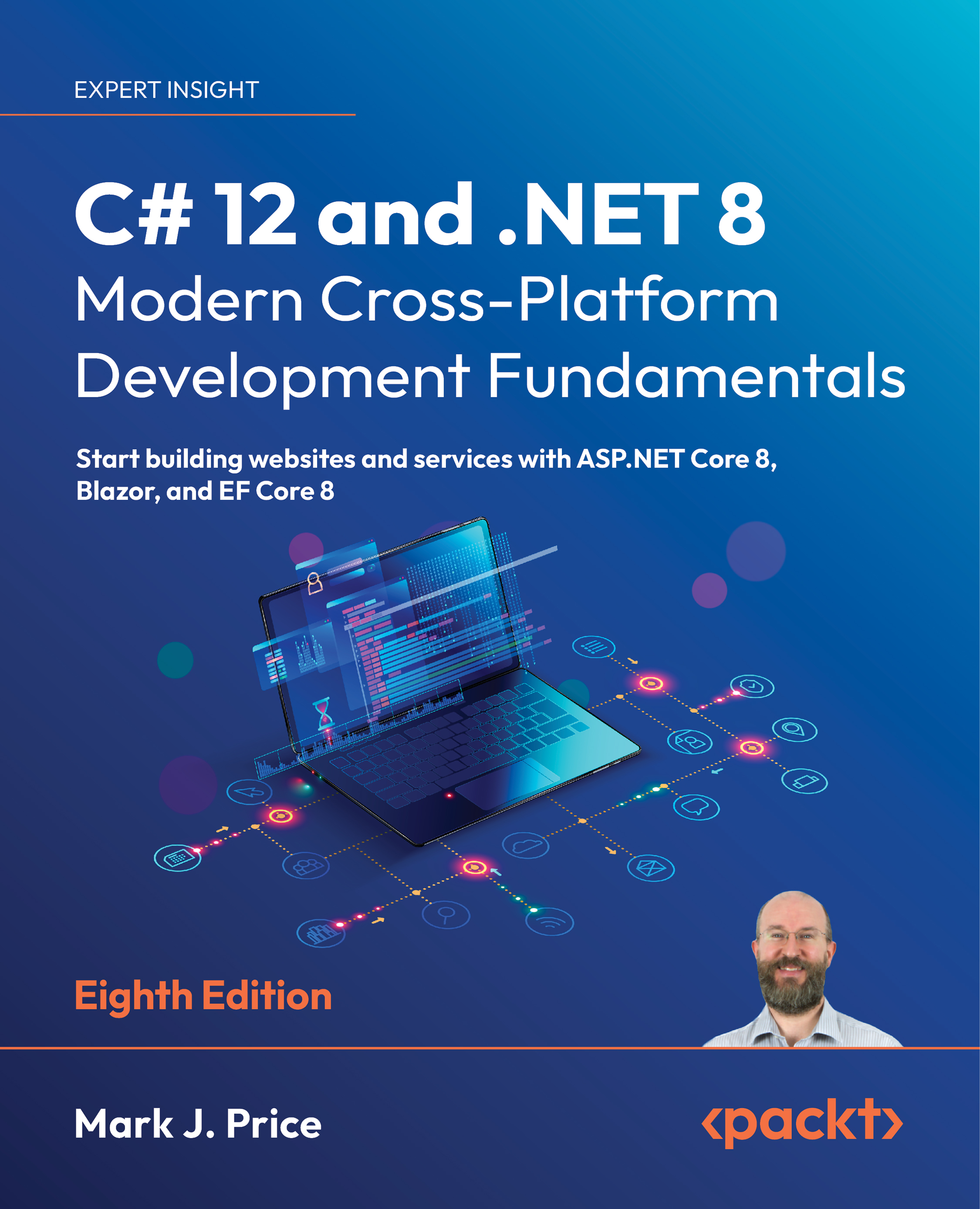
C# 12 and .NET 8 – Modern Cross-Platform Development Fundamentals
By :
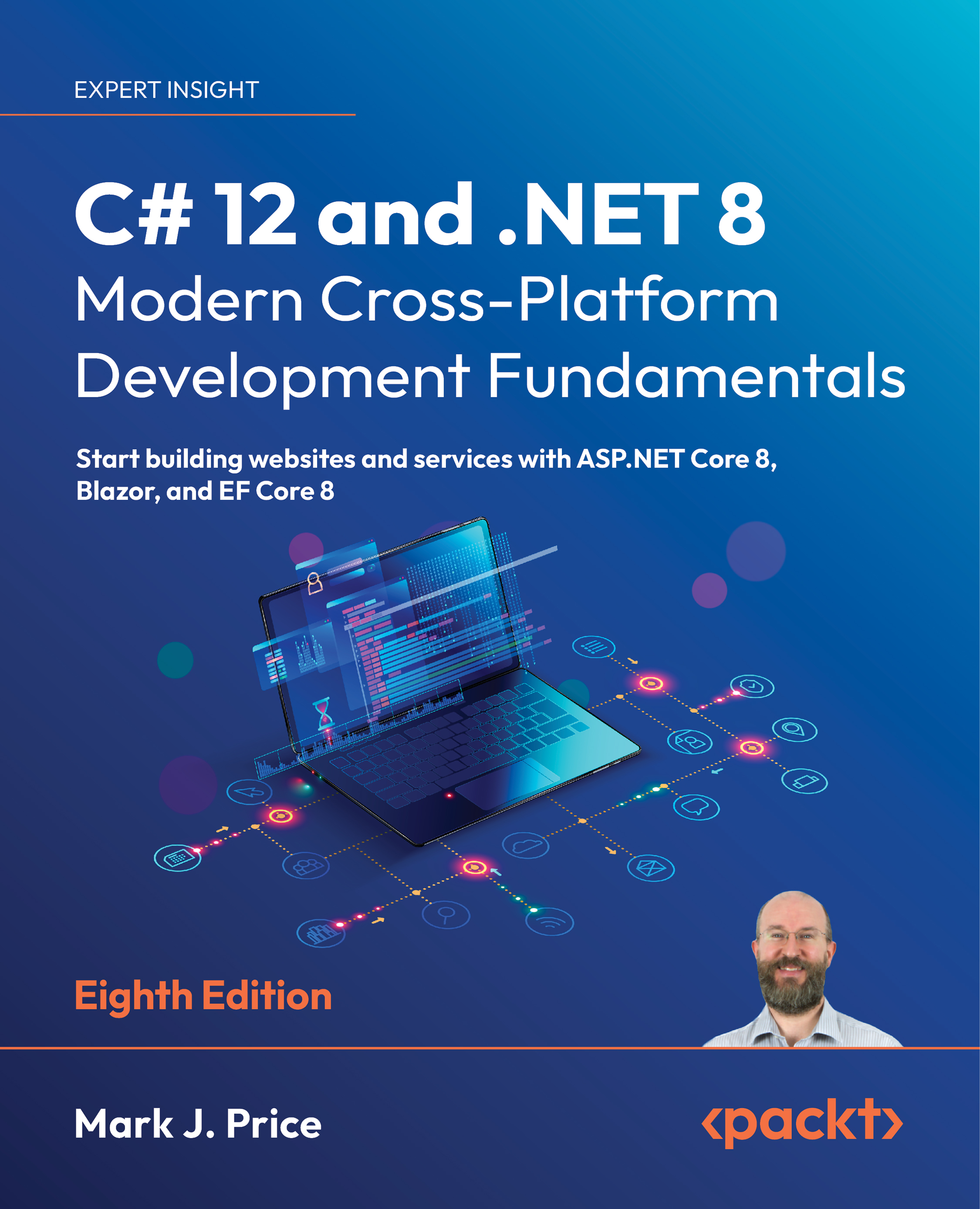
C# 5 introduced two C# keywords when working with the Task
type that enable easy multithreading. The pair of keywords is especially useful for the following:
In an online section, Building Websites Using the Model-View-Controller Pattern, we will see how the async
and await
keywords can improve scalability for websites. But for now, let’s see an example of how they can be used in a console app, and then later you will see them used in a more practical example within web projects.
One of the limitations with console apps is that you can only use the await
keyword inside methods that are marked as async
, but C# 7 and earlier do not allow the Main
method to be marked as async
! Luckily, a new feature introduced in C# 7.1 was support for async
in Main
.
Let’s see it in action:
console
project named AsyncConsole
to the Chapter02
solution.AsyncConsole.csproj
, and after the <PropertyGroup>
section, add a new <ItemGroup>
section to statically import System.Console
for all C# files using the implicit usings .NET SDK feature, as shown in the following markup:
<ItemGroup>
<Using Include="System.Console" Static="true" />
</ItemGroup>
Program.cs
, delete the existing statements, and then add statements to create an HttpClient
instance, make a request for Apple’s home page, and output how many bytes it has, as shown in the following code:
HttpClient client = new();
HttpResponseMessage response =
await client.GetAsync("http://www.apple.com/");
WriteLine("Apple's home page has {0:N0} bytes.",
response.Content.Headers.ContentLength);
In .NET 5 and earlier, you would have seen an error message, as shown in the following output:
Program.cs(14,9): error CS4033: The 'await' operator can only be used within an async method. Consider marking this method with the 'async' modifier and changing its return type to 'Task'. [/Users/markjprice/Code/ Chapter02/AsyncConsole/AsyncConsole.csproj]
You would have had to add the async
keyword for your Main
method and change its return type from void
to Task
. With .NET 6 and later, the console app project template uses the top-level program feature to automatically define the Program
class with an asynchronous <Main>$
method for you.
Apple's home page has 170,688 bytes.