-
Book Overview & Buying
-
Table Of Contents
-
Feedback & Rating
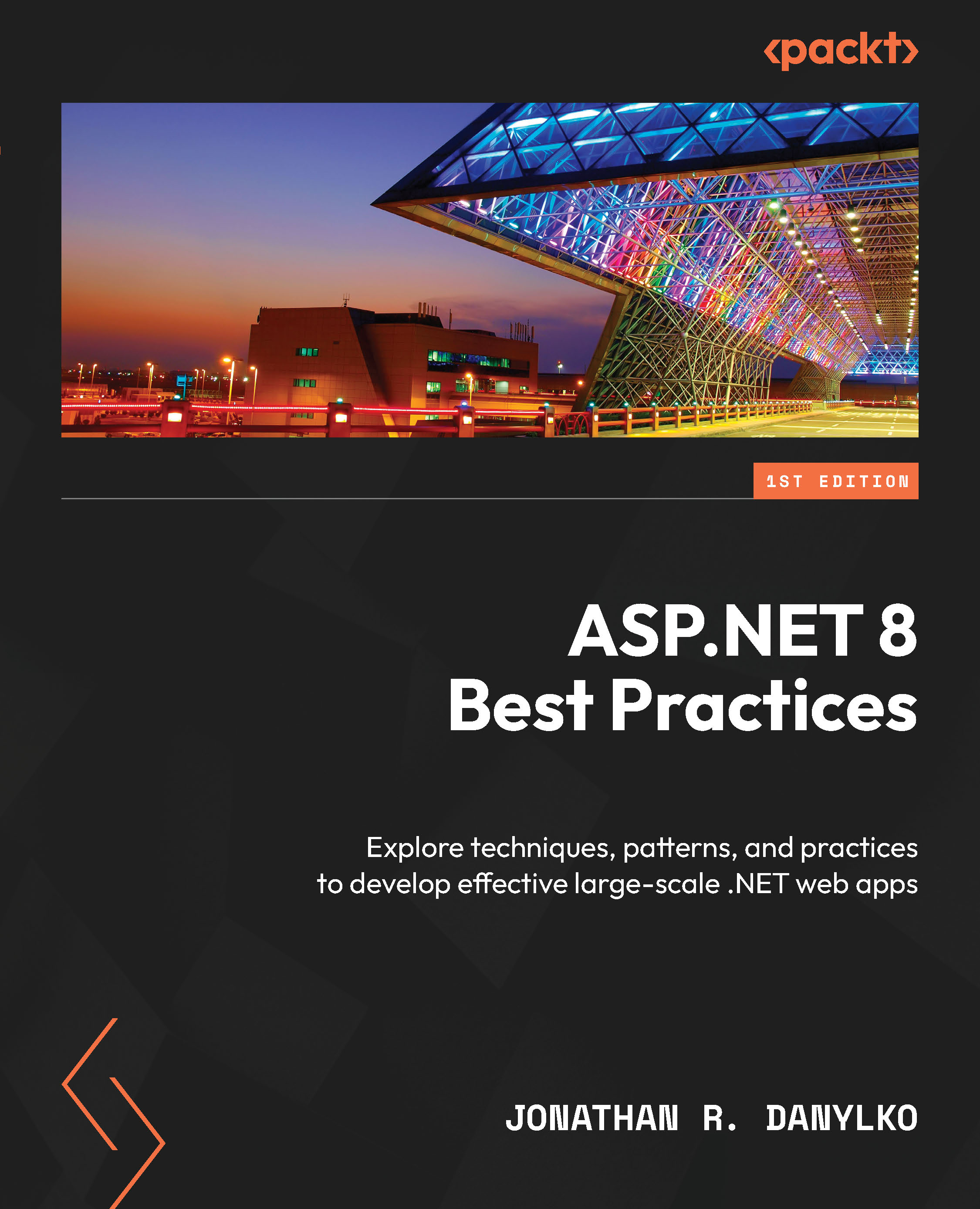
ASP.NET 8 Best Practices
By :
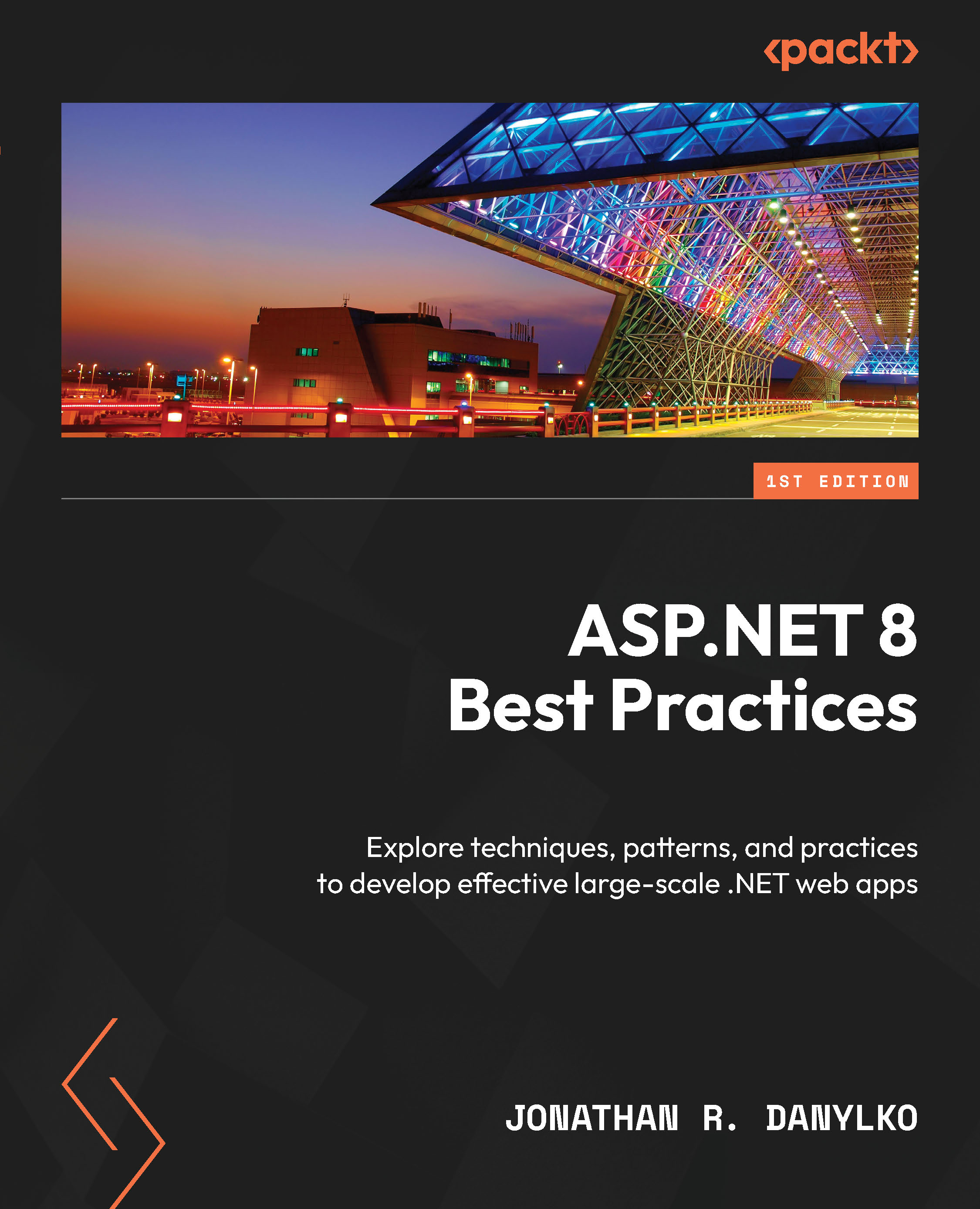
Deploying application code is one thing but deploying databases can be a daunting task if not done properly. There are two pain points when deploying databases: structure and records.
With a database’s structure, you have the issue of adding, updating, and removing columns/fields from tables, along with updating the corresponding stored procedures, views, and other table-related functions to reflect the table updates.
With records, the process isn’t as tricky as changing a table’s structure. The frequency of updating records is not as regular, but when it does, happen that’s when you either want to seed a database with default records or update those seed records with new values.
The following sections will cover some common practices when deploying databases in a CI/CD pipeline.
Since company data is essential to a business, it’s mandatory to back it up before making any modifications or updates to the database.
One recommendation is to make the entire database deploy a two-step process: back up the database, then apply the database updates.
The DevOps team can include a pre-deployment script to automatically back up the database before applying the database updates. If the backup was successful, you can continue deploying your changes to the database. If not, you can immediately stop the deployment and determine the cause of failure.
As discussed in the previous section, this is necessary for a “fallback” approach instead of a “fall forward” strategy.
One strategy for updating a table is to take a non-destructive approach:
While making the appropriate changes to table structures, don’t forget about updating the additional database code to reflect the table changes, including stored procedures, views, and functions.
If your Visual Studio solution connects to a database, there’s another project type you need to add to your solution called the Database Project type. When you add this project to your solution, it takes a snapshot of your database and adds it to your project as code.
Why include this in your solution? There are three reasons to include it in your solution:
As you can see, it’s pretty handy to include with your solution.
Entity Framework has come a long way since its early days. Migrations are another way to include database changes through C# as opposed to T-SQL.
Upon creating a migration, Entity Framework Core takes a snapshot of the database and DbContext
and creates the delta between the database schema and DbContext
using C#.
With the initial migration, the entire C# code is generated with an Up()
method.
Any subsequent migrations will contain an Up()
method and a Down()
method for upgrading and downgrading the database, respectively. This allows developers to save their database delta changes, along with their code changes.
Entity Framework Core’s migrations are an alternative to using DACs and custom scripts. These migrations can perform database changes based on the C# code.
If you require seed records, then you can use Entity Framework Core’s .HasData()
method for easily creating seed records for tables.
In this section, we learned how to prepare our database deployment by always creating a backup, looked at a common strategy for adding, updating, and deleting table fields, and learned how to deploy databases in a CI/CD pipeline using either a DAC or Entity Framework Core’s migrations.