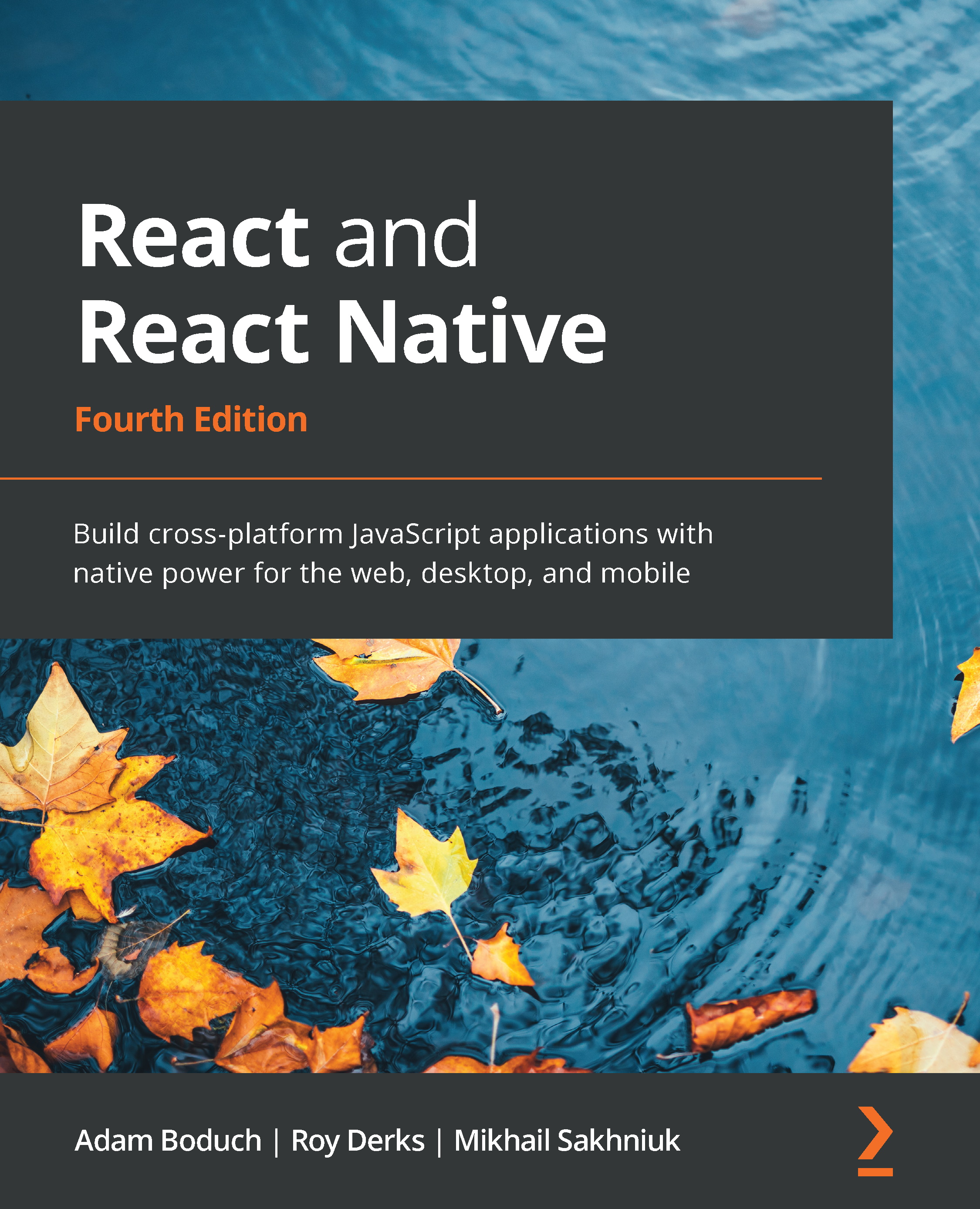
React and React Native
By :
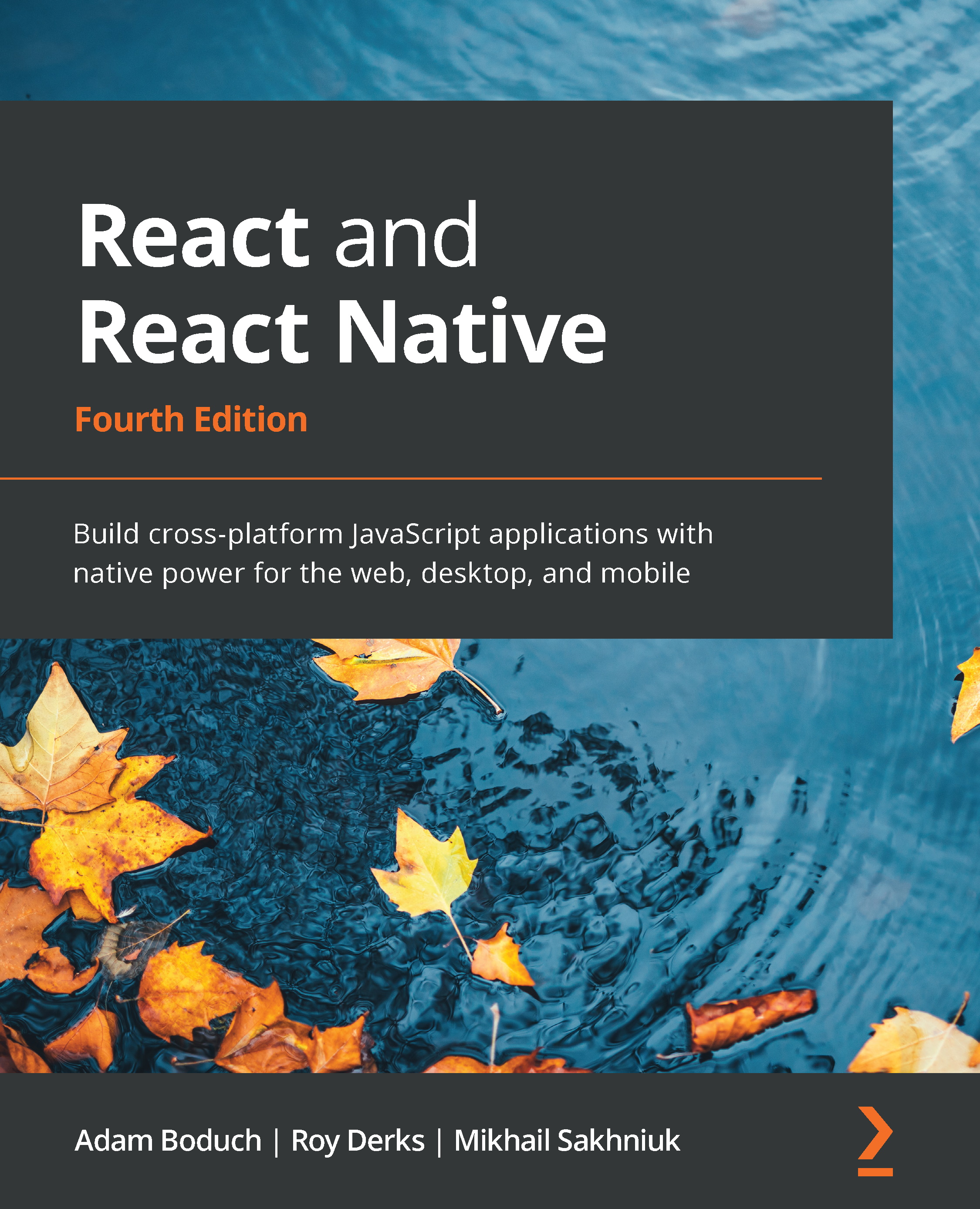
Chapter 1, Why React?, describes what React is and why you want to use it to build your application.
Chapter 2, Rendering with JSX, teaches the basics of JSX, the markup language used by React components.
Chapter 3, Context Properties, State, and Context, introduces the core mechanisms of passing data around your React application.
Chapter 4, Getting Started with Hooks, shows how React Hooks can be used to extend the behavior of components.
Chapter 5, Event Handling, the React Way, gives an overview of how events are handled by React components.
Chapter 6, Crafting Reusable Components, guides you through the process of refactoring components by example.
Chapter 7, The React Component Life Cycle, describes the various phases that React components go through and why it's important for React developers.
Chapter 8, Validating Component Properties, shows you how to ensure that React component property values are as expected.
Chapter 9, Handling Navigations with Routes, provides plenty of examples of how to set up routing for your React web app.
Chapter 10, Code Splitting Using Lazy Components and Suspense, introduces code-splitting techniques that result in smaller, more efficient applications.
Chapter 11, Server-Side React Components, teaches you how to use Next.js to build large-scale React applications that render content on a server and a client.
Chapter 12, User Interface Framework Components, gives an overview of how to get started with MUI, a React component library for building UIs.
Chapter 13, High-Performance State Updates, goes into depth on the new features in React 18 that allow for efficient state updates and a high-performing application.
Chapter 14, Why React Native?, describes what the React Native library is and the differences between native mobile development.
Chapter 15, React Native under the Hood, gives an overview of the architecture of React Native.
Chapter 16, Kick-Starting React Native Projects, teaches you how to start a new React Native project.
Chapter 17, Building Responsive Layouts with Flexbox, describes how to create a layout and add styles.
Chapter 18, Navigating between Screens, shows the approaches to switching between screens in an app.
Chapter 19, Rendering Item Lists, describes how to implement lists of data in an application.
Chapter 20, Showing Progress, shows you how to handle process indications and progress bars.
Chapter 21, Geolocation and Maps, guides you on how to track geolocation and add a map to an app.
Chapter 22, Collecting User Input, teaches you how to create forms.
Chapter 23, Displaying Modal Screens, teaches you how to create dialog modals.
Chapter 24, Responding to User Gestures, provides examples of how to handle user gestures.
Chapter 25, Using Animations, describes how to implement animations in an app.
Chapter 26, Controlling Image Display, gives an overview of how to render images in a React Native app.
Chapter 27, Going Offline, shows how to deal with an app when a mobile phone doesn't have an internet connection.
Chapter 28, Selecting Native UI Components Using NativeBase, teaches you how to create an application using the NativeBase UI library.
Chapter 29, Handling Application State, shows you how to handle application state for both web and mobile apps.
Chapter 30, Why GraphQL?, describes what GraphQL is and how to use it.
Chapter 31, Building a React GraphQL App, shows how to handle GraphQL in React and React Native apps.