-
Book Overview & Buying
-
Table Of Contents
-
Feedback & Rating
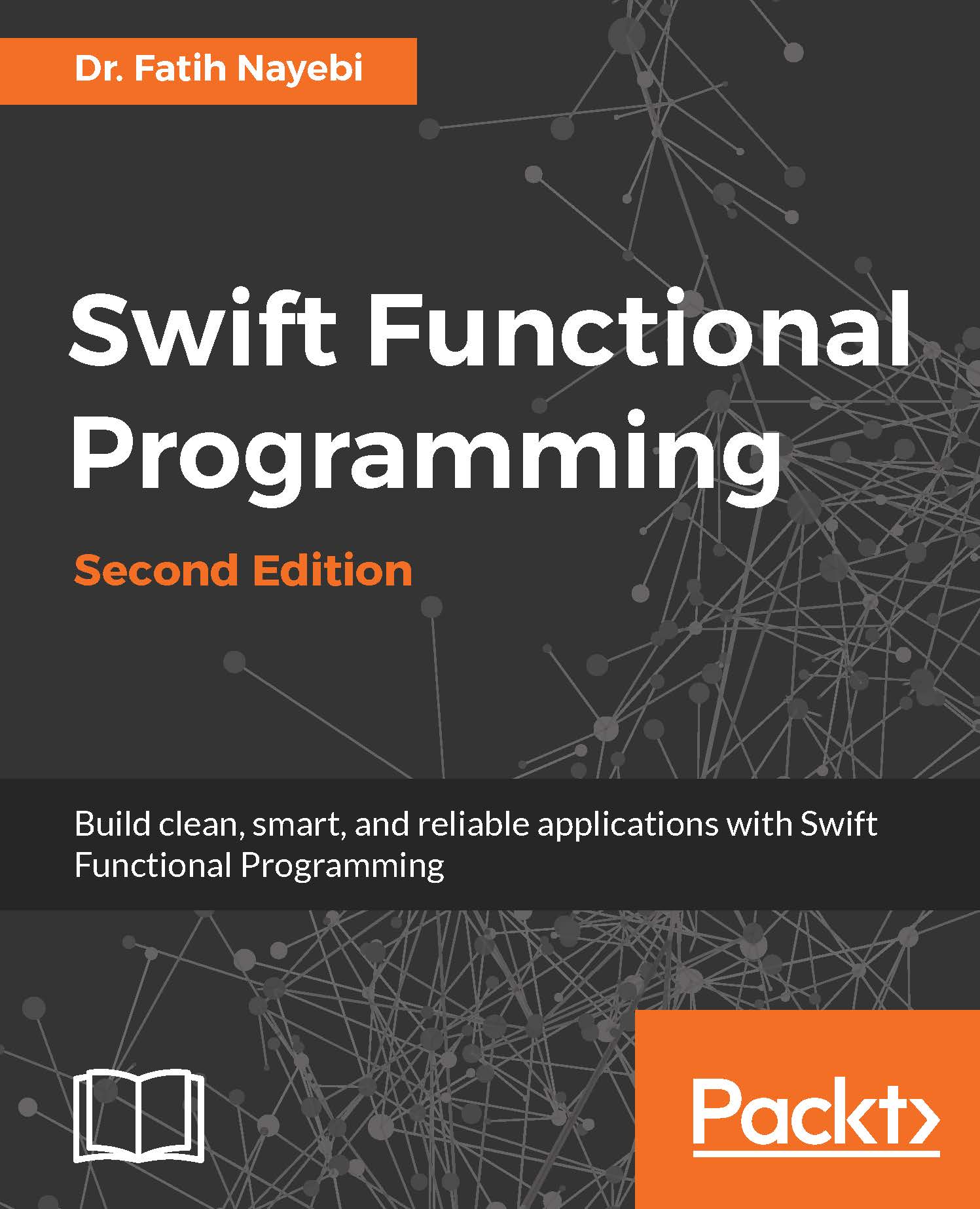
Swift Functional Programming
By :
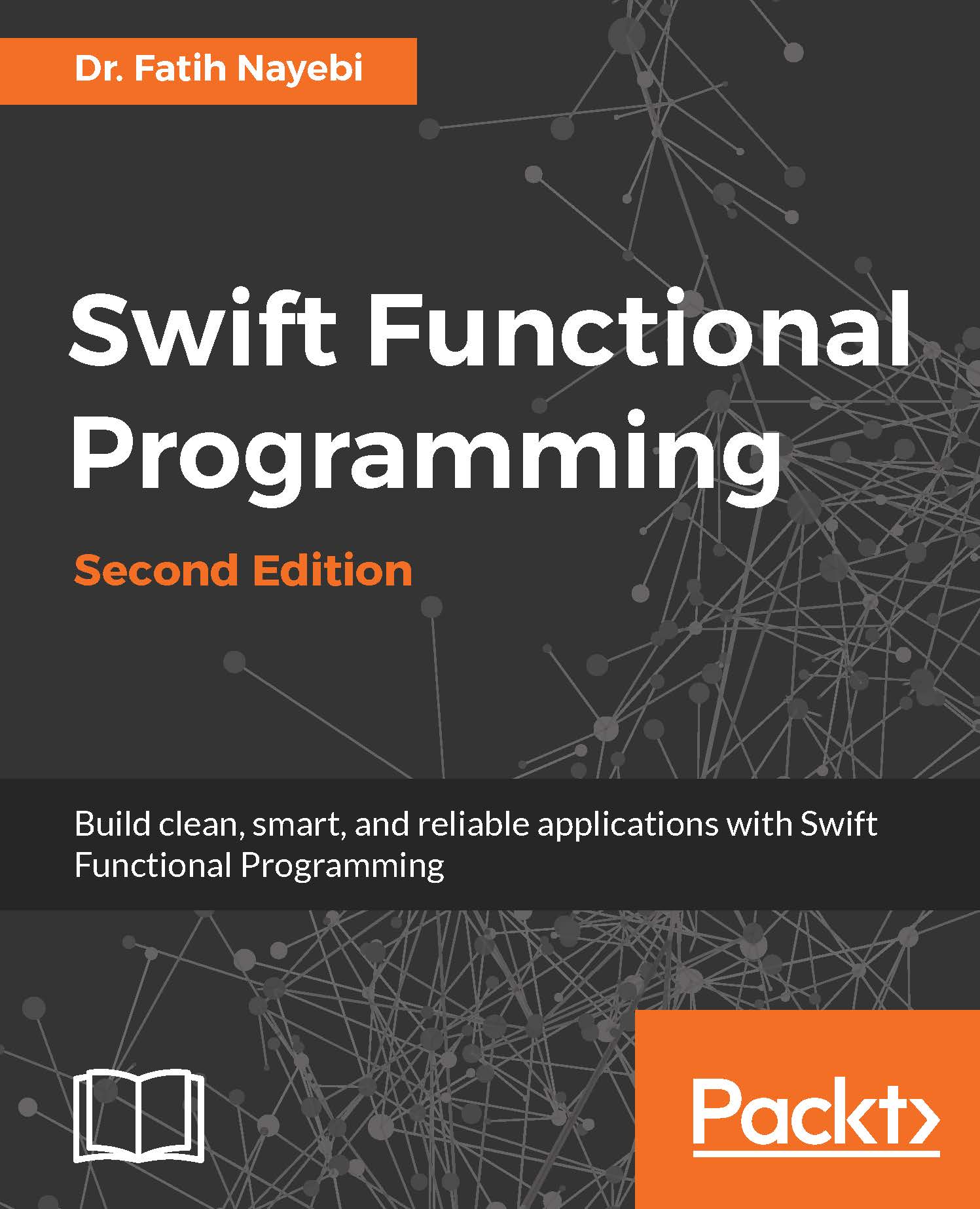
flatMap
is a generic instance method for any type that conforms to Sequence
protocol. It can be used to flatten one level of a dimension of a sequence or to remove nil values in the sequence. The following example presents a two-dimensional array, in other words, nested arrays.
Calling flatMap
on this array reduces one dimension and flattens it so the resulting array becomes [1, 3, 5, 2, 4, 6]
:
let twoDimArray = [[1, 3, 5], [2, 4, 6]] let oneDimArray = twoDimArray.flatMap { $0 }
In this example, flatMap
returns an array containing the concatenated results of the mapping transform over itself. We can achieve the same result by calling joined
on our array and then map
, as follows:
let oneDimArray = twoDimArray.joined().map { $0 }
To be able to transform each element in an array, we will need to provide a map
method as the closure to the flatMap
method as follows:
let transofrmedOneDimArray = twoDimArray.flatMap { $0.map { $0 + 2 } }
The result will be [3, 5, 7, 4, 6, 8]
.
The...