-
Book Overview & Buying
-
Table Of Contents
-
Feedback & Rating
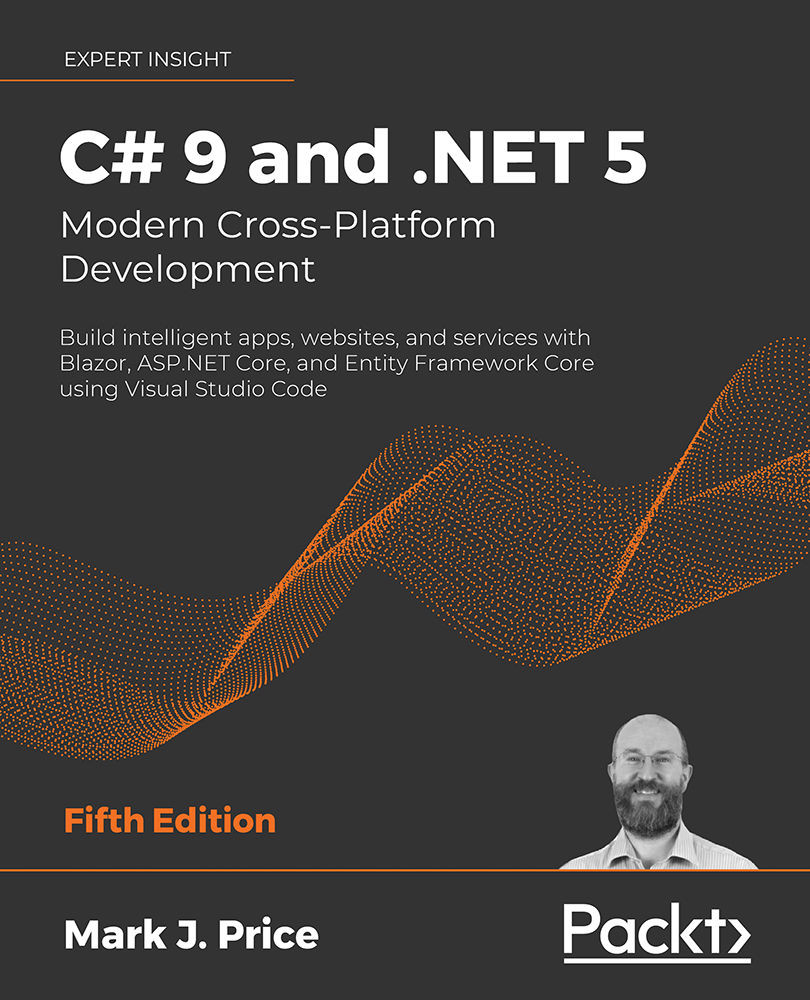
C# 9 and .NET 5 – Modern Cross-Platform Development
By :
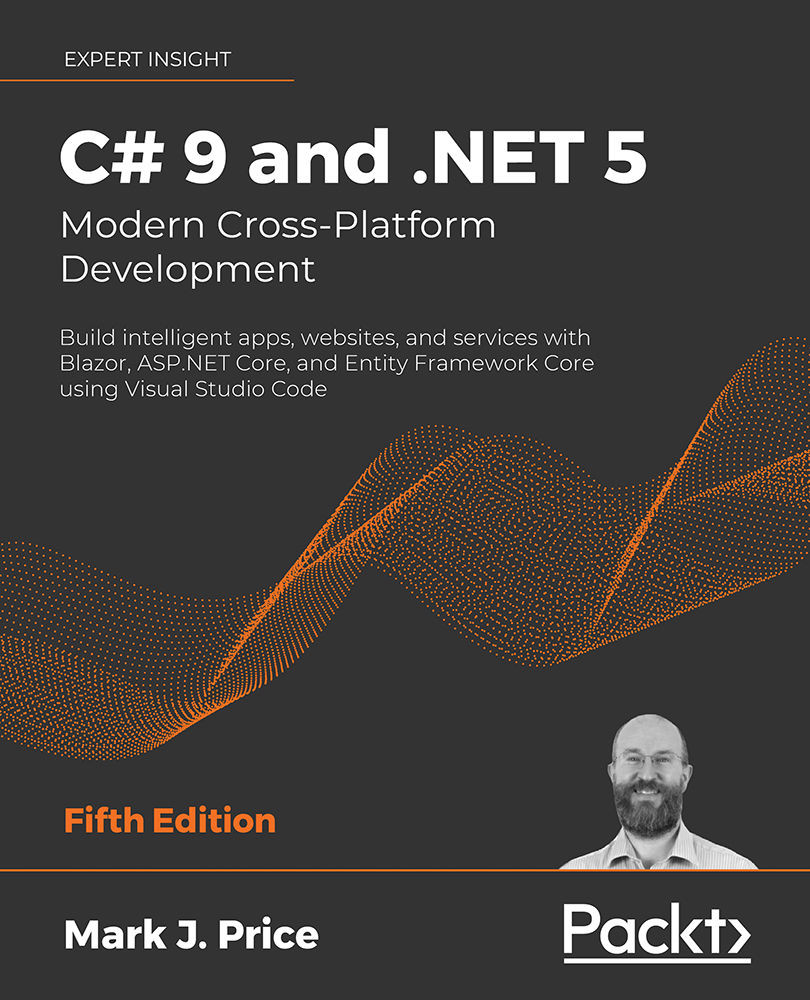
Every application needs to be able to select from choices and branch along different code paths. The two selection statements in C# are if
and switch
. You can use if
for all your code, but switch
can simplify your code in some common scenarios such as when there is a single variable that can have multiple values that each require different processing.
The if
statement determines which branch to follow by evaluating a Boolean expression. If the expression is true
, then the block executes. The else
block is optional, and it executes if the if
expression is false
. The if
statement can be nested.
The if
statement can be combined with other if
statements as else if
branches, as shown in the following code:
if (expression1)
{
// runs if expression1 is true
}
else if (expression2)
{
// runs if expression1 is false and expression2 if true
}
else if (expression3)
{
// runs if expression1 and expression2...