-
Book Overview & Buying
-
Table Of Contents
-
Feedback & Rating
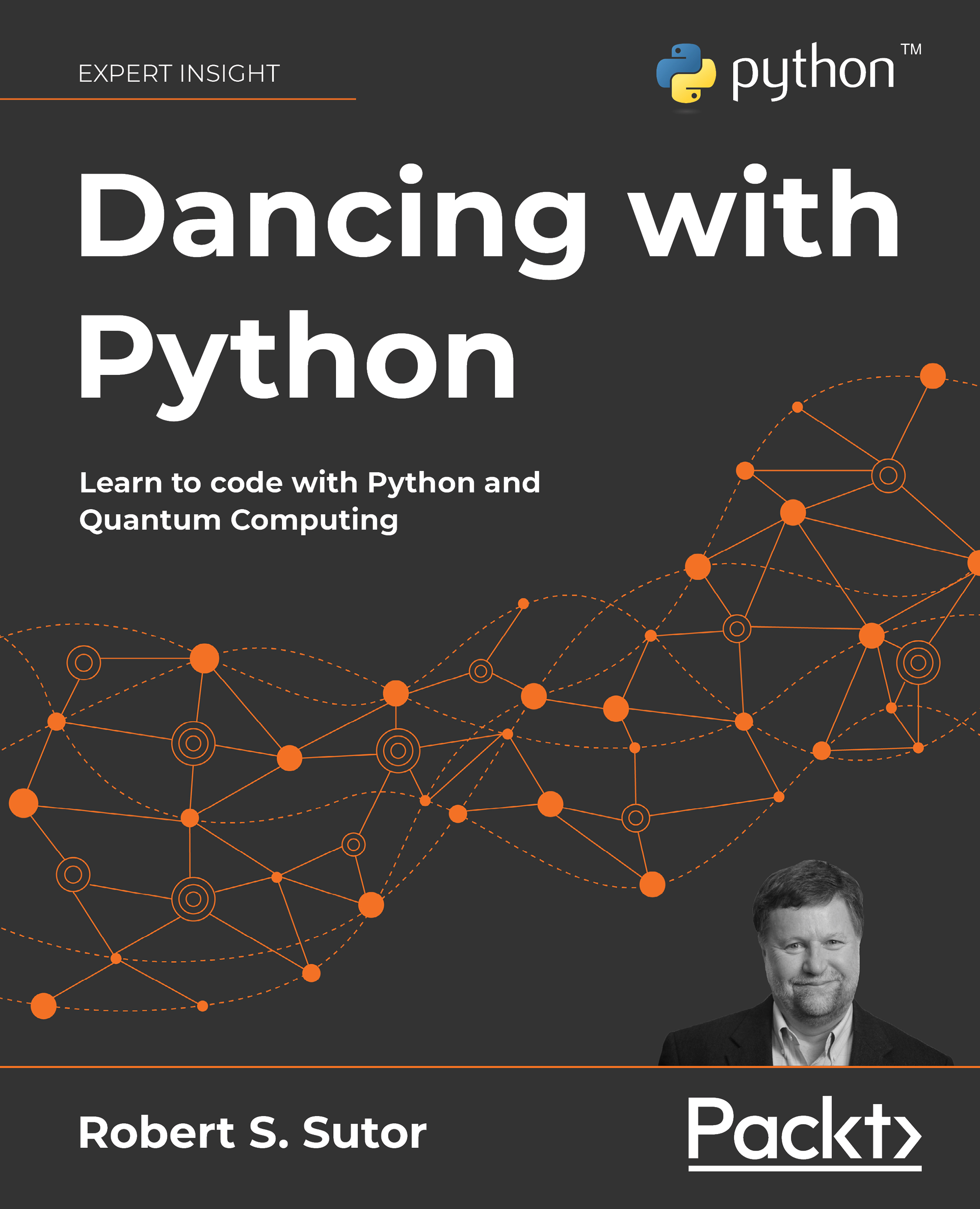
Dancing with Python
By :
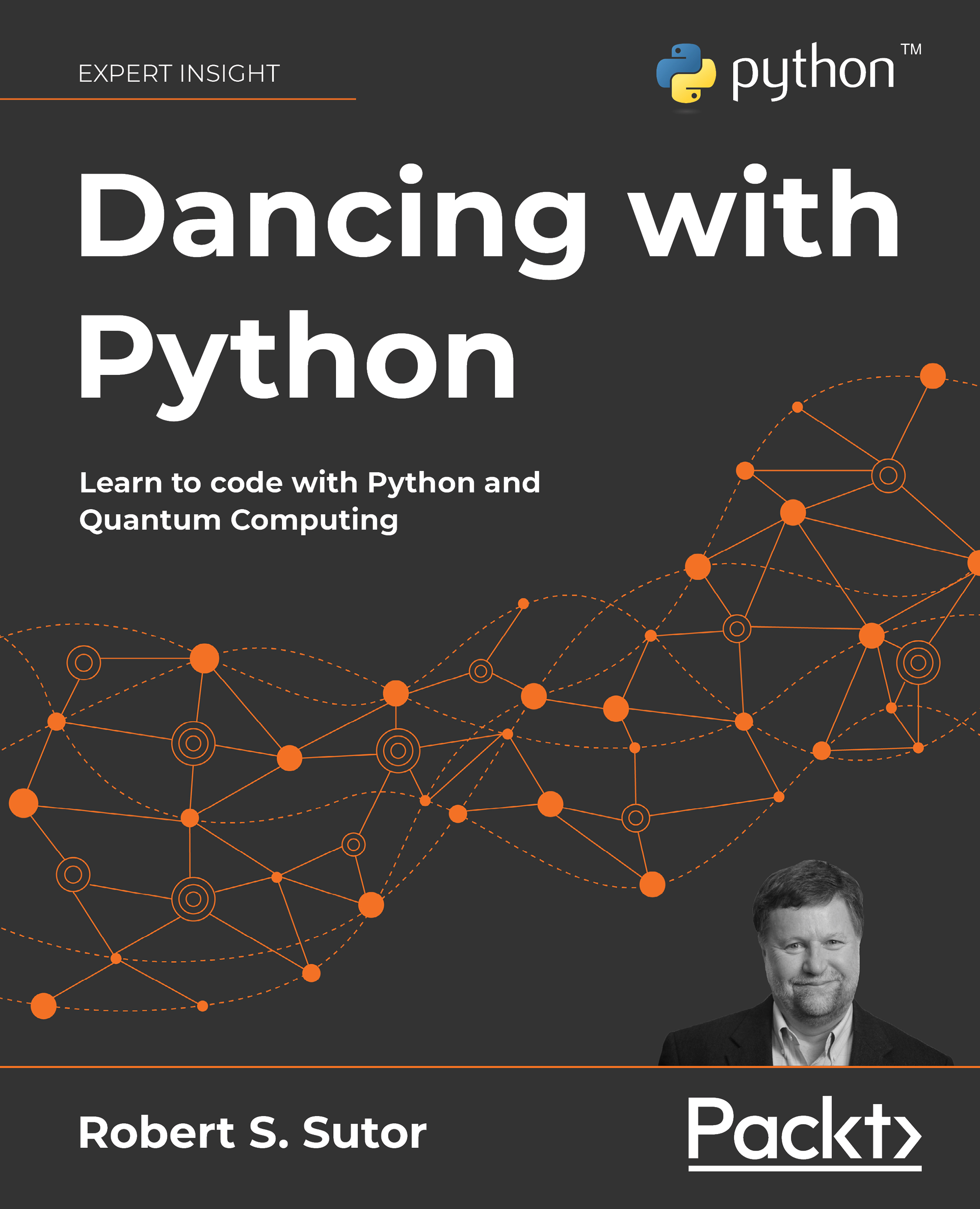
The notion of “expression” includes functions and all that goes into writing and using them. The syntax of their definition varies significantly among programming languages, unlike simple arithmetic expressions. In words, I can say
The function maximum(x, y)
returns x
if it is larger than y. Otherwise, it returns y
.
Let’s consider this informal definition.
x
and y
. These are
the parameters of the function.maximum(3, -2)
to get the larger value, we
call the function maximum on the arguments
3
and -2
.x
and y
are parameters, and 3
and
-2
are arguments. However, it’s not unusual for coders to call them all
arguments.x
larger than y
” is the inequality x >
y
. This returns a Boolean true or false. Some languages use “predicate”
to refer to an expression that returns a Boolean.For comparison, this is one way of writing maximum in the C programming language:
int maximum(int x, int y) {
if (x > y) {
return x;
}
return y;
}
This is a Python version with a similar definition:
def maximum(x, y):
if x > y:
return x
return y
There are several variations within each language for accomplishing the same result. Since they look so similar in different languages, you should always ask, “Am I doing this the best way in this programming language?”. If not, why are you using this language instead of another?
Expressions can take up several lines, particularly function definitions. C uses braces
“{ }
” to group parts together, while Python uses indentation.