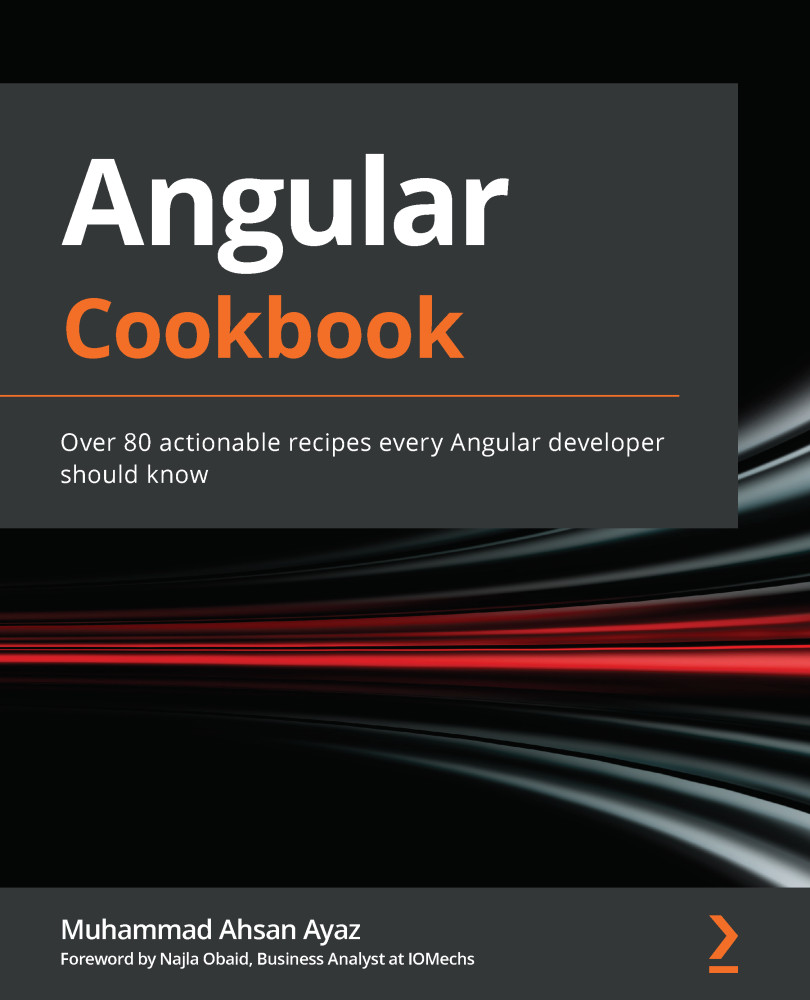
Angular Cookbook
By :
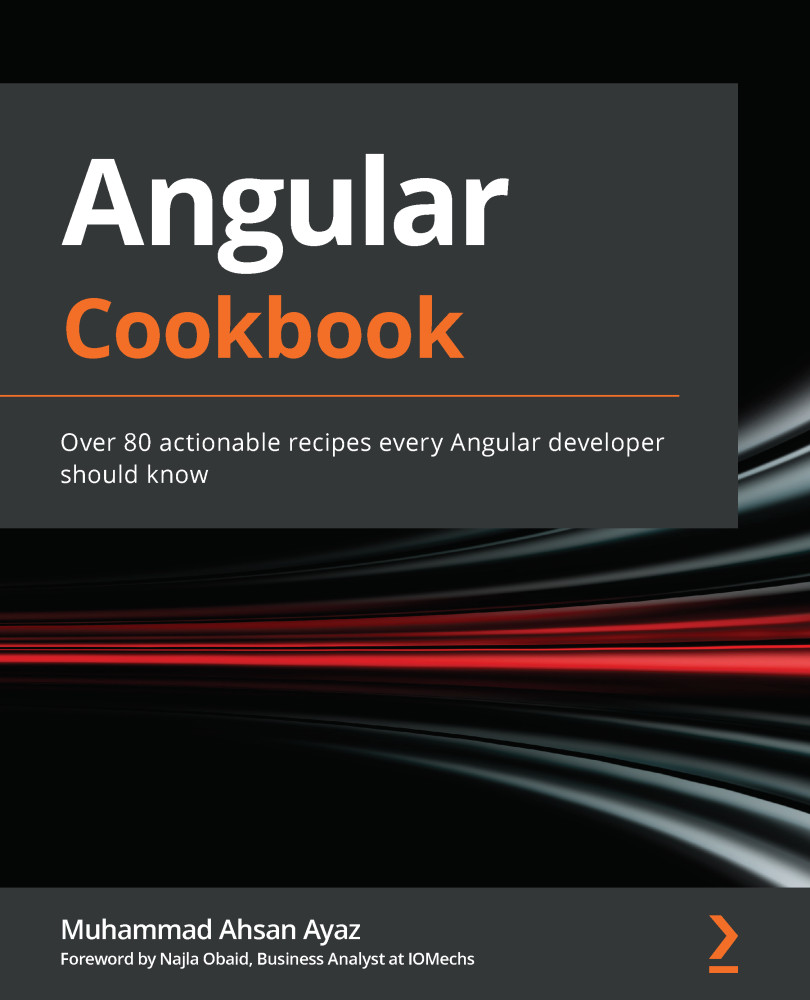
In this recipe, you'll learn how to improve type checking in templates for your custom Angular directives using the static template guards that the recent versions of Angular have introduced. We'll enhance the template type checking for our appHighlight
directive so that it only accepts a narrowed set of inputs.
The project we are going to work with resides in chapter02/start_here/enhanced-template-type-checking
, inside the cloned repository:
npm install
to install the dependencies of the project. ng serve -o
. This should open the app in a new browser tab, and you should see something like this:
Figure 2.8 – enhanced-template-type-checking app running on http://localhost:4200
Now that we have the app running, let's see the steps for this recipe in the next section.
highlightColor
attribute/input for the appHighlight
directive. Give it a try. Provide the '#dcdcdc'
value as the input and you'll have a broken highlight color, but no errors whatsoever:... <div class="content" role="main"> ... <p class="text-content" appHighlight [highlightColor]="'#dcdcdc'" [highlightText]="searchText"> ... </p> </div>
angularCompileOptions
to our tsconfig.json
file. We'll do this by adding a flag named strictInputTypes
as true
. Stop the app server, modify the code as follows, and rerun the ng serve
command to see the changes:{ "compileOnSave": false, "compilerOptions": { ... }, "angularCompilerOptions": { "strictInputTypes": true } }
You should see something like this:
Figure 2.9 – strictInputTypes helping with build time errors for incompatible type
'#dcdcdc'
value is not assignable to the HighlightColor
type. But what happens if someone tries to provide null
as the value? Would it still be fine? The answer is no. We would still have a broken experience, but no error whatsoever. To fix this, we'll enable two flags for our angularCompilerOptions
—strictNullChecks
and strictNullInputTypes
:{ "compileOnSave": false, "compilerOptions": { ... }, "angularCompilerOptions": { "strictInputTypes": true, "strictNullChecks": true, "strictNullInputTypes": true } }
app.component.html
file to provide null
as the value for the [highlightColor]
attribute, as follows:... <div class="content" role="main"> ... <p class="text-content" appHighlight [highlightColor]="null" [highlightText]="searchText"> ... </div>
ng serve
, and you'll see that we now have another error, as shown here:Figure 2.10 – Error reporting with strictNullInputTypes and strictNullChecks in action
strictNullChecks
flag and the strictTemplates
flag:{ "compileOnSave": false, "compilerOptions": { ... }, "angularCompilerOptions": { "strictNullChecks": true, "strictTemplates": true } }
HighlightColor
enum into our app.component.ts
file. We will add a hColor
property to the AppComponent
class and will assign it a value from the HighlightColor
enum, as follows:import { Component } from '@angular/core'; import { HighlightColor } from './directives/highlight.directive'; @Component({ selector: 'app-root', templateUrl: './app.component.html', styleUrls: ['./app.component.scss'] }) export class AppComponent { searchText = ''; hColor: HighlightColor = HighlightColor.LightCoral; }
hColor
property in the app.component.html
file to pass it to the appHighlight
directive. This should fix all the issues and make light coral the assigned highlight color for our directive:<div class="content" role="main"> ... <p class="text-content" appHighlight [highlightColor]="hColor" [highlightText]="searchText"> ... </p> </div>