-
Book Overview & Buying
-
Table Of Contents
-
Feedback & Rating
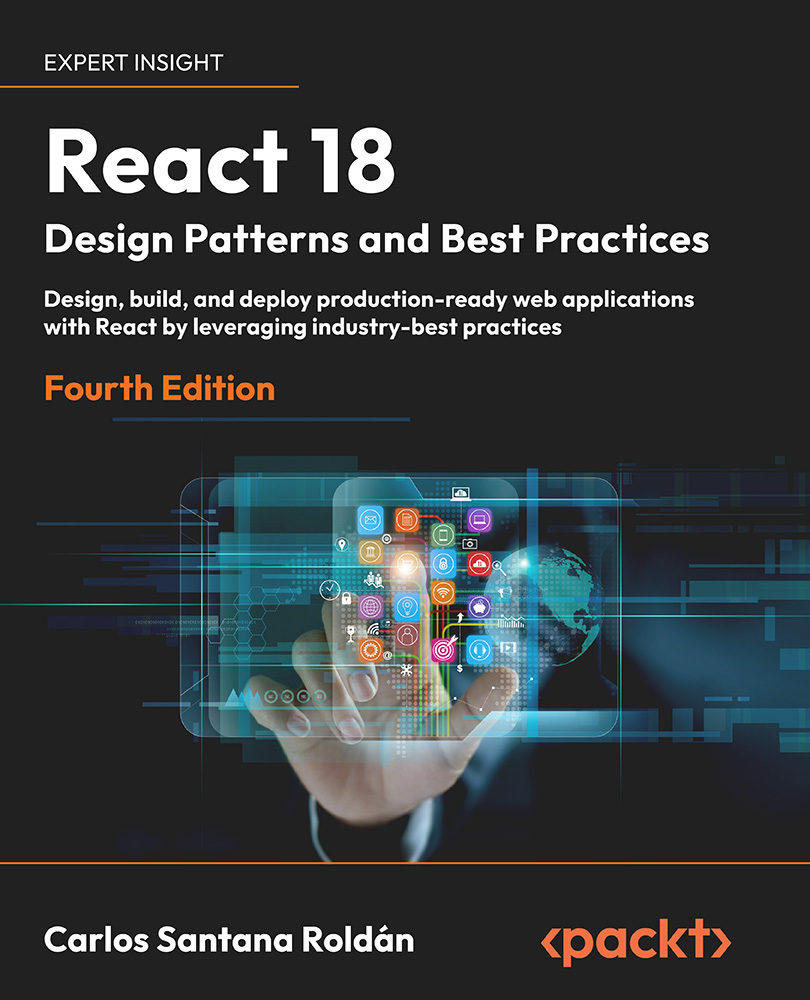
React 18 Design Patterns and Best Practices
By :
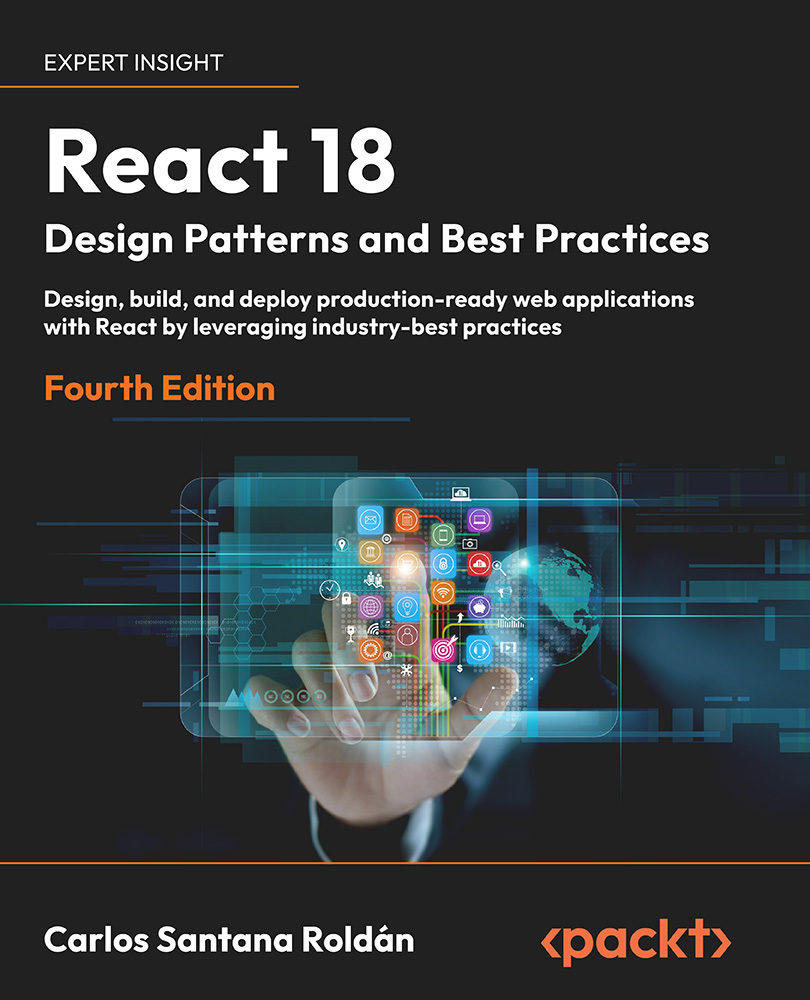
In this section, we will see how to transform some JavaScript code into TypeScript.
Let’s suppose we have to check whether a word is a palindrome. The JavaScript code for this algorithm will be as follows:
function isPalindrome(word) {
const lowerCaseWord = word.toLowerCase()
const reversedWord = lowerCaseWord.split('').reverse().join('')
return lowerCaseWord === reversedWord
}
You can name this file palindrome.ts
.
As you can see, we are receiving a string
variable (word
), and we are returning a boolean
value. So, how will this be translated into TypeScript?
function isPalindrome(word: string): boolean {
const lowerCaseWord = word.toLowerCase()
const reversedWord = lowerCaseWord.split('').reverse().join('')
return lowerCaseWord === reversedWord
}
You’re probably thinking, “Great, I just specified the string
type as word
and the boolean
type to the function returned value, but now what?”
If you try to run the function with some value that is different from string
, you will get a TypeScript error:
console.log(isPalindrome('Level')) // true
console.log(isPalindrome('Anna')) // true
console.log(isPalindrome('Carlos')) // false
console.log(isPalindrome(101)) // TS Error
console.log(isPalindrome(true)) // TS Error
console.log(isPalindrome(false)) // TS Error
So, if you try to pass a number to the function, you will get the following error:
Figure 2.1: Type number is not assignable to parameter of type string
That’s why TypeScript is very useful, because it will force you to be stricter and more explicit with your code.