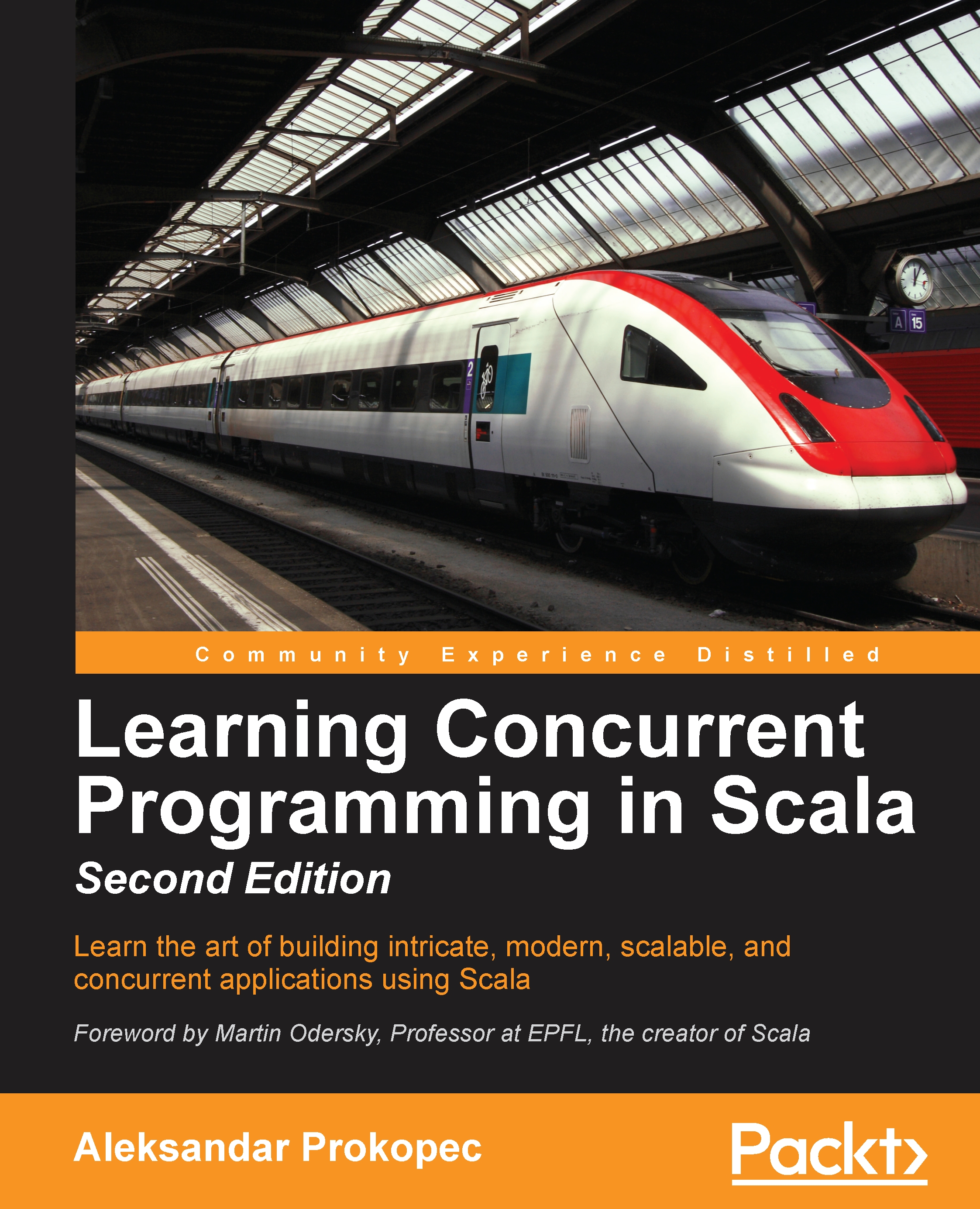
Learning Concurrent Programming in Scala
By :
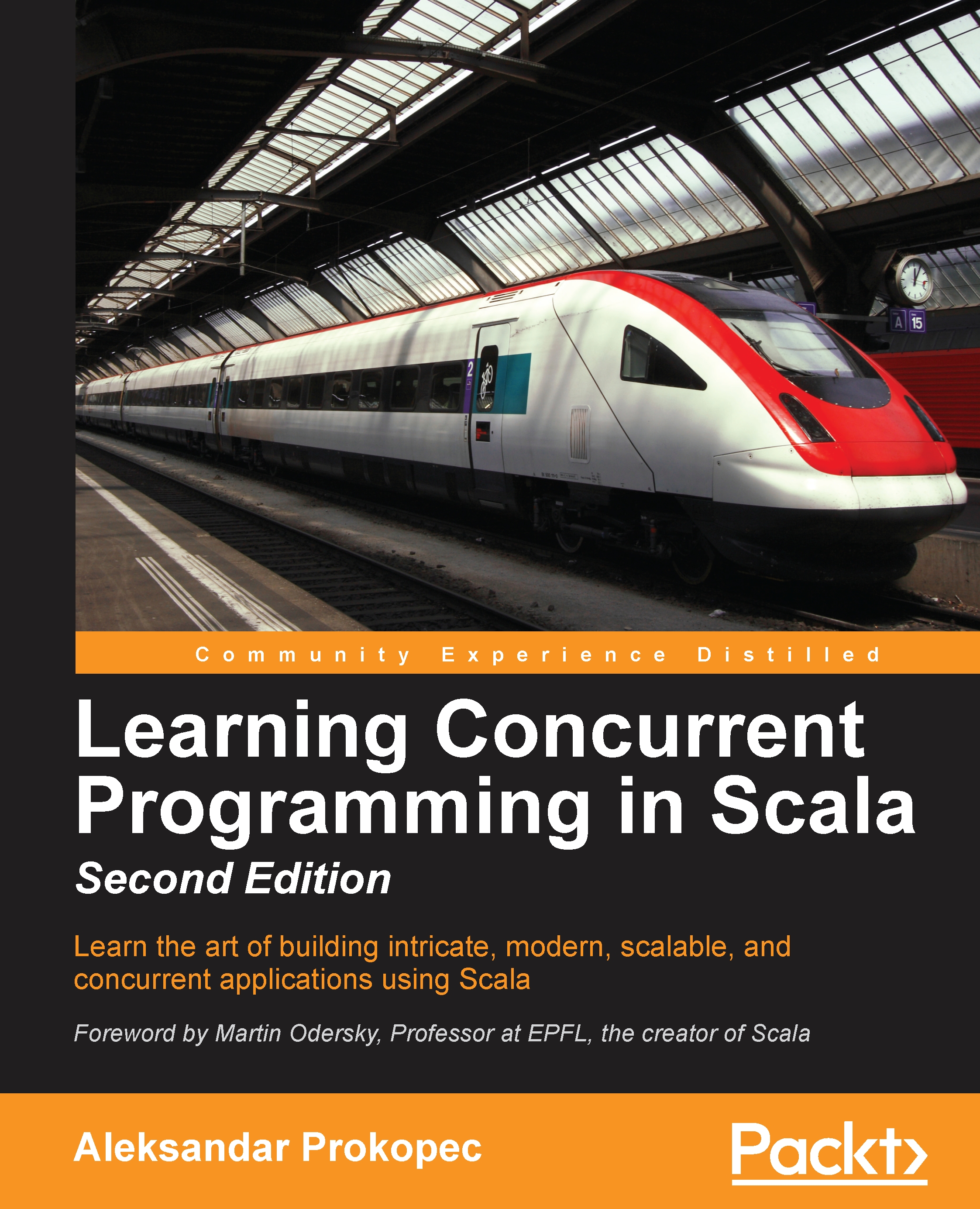
The following exercises are designed to test your knowledge of the Scala programming language. They cover the content presented in this chapter, along with some additional Scala features. The last two exercises contrast the difference between concurrent and distributed programming, as defined in this chapter. You should solve them by sketching out a pseudocode solution, rather than a complete Scala program.
compose
method with the following signature:def compose[A, B, C] (g: B => C, f: A => B): A => C = ???
This method must return a function h
, which is the composition of the functions f
and g
fuse
method with the following signature:def fuse[A, B] (a: Option[A], b: Option[B]): Option[(A, B)] = ???
The resulting Option
object should contain a tuple of values from the Option
objects a
and b
, given that both a
and b
are non-empty. Use for-comprehensions
check
method, which takes a set of values of type T
and a function of type T => Boolean
:def check[T](xs: Seq[T])(pred: T => Boolean): Boolean = ???
The method must return true
if and only if the pred
function returns true
for all the values in xs
without throwing an exception. Use the check
method as follows:
check(0 until 10)(40 / _ > 0)
The check
method has a curried definition: instead of just one parameter list, it has two of them. Curried definitions allow a nicer syntax when calling the function, but are otherwise semantically equivalent to single-parameter list definitions.
Pair
class from this chapter so that it can be used in a pattern match.If you haven't already done so, familiarize yourself with pattern matching in Scala.
permutations
function, which, given a string, returns a sequence of strings that are lexicographic permutations of the input string:def permutations(x: String): Seq[String]
combinations
function that, given a sequence of elements, produces an iterator over all possible combinations of length n
. A combination is a way of selecting elements from the collection so that every element is selected once, and the order of elements does not matter. For example, given a collection Seq(1, 4, 9, 16)
, combinations of length 2 are Seq(1, 4)
, Seq(1, 9)
, Seq(1, 16)
, Seq(4, 9)
, Seq(4, 16)
, and Seq(9, 16)
. The combinations function has the following signature:def combinations(n: Int, xs: Seq[Int]): Iterator[Seq[Int]]
See the Iterator
API in the standard library documentation
def matcher (regex: String): PartialFunction[String, List[String]]
The partial function should not be defined if there are no matches within the argument strings. Otherwise, it should use the regular expression to output the list of matches.
Solve the problem from the previous exercise, this time using the whiteboard.
Change the font size
Change margin width
Change background colour