-
Book Overview & Buying
-
Table Of Contents
-
Feedback & Rating
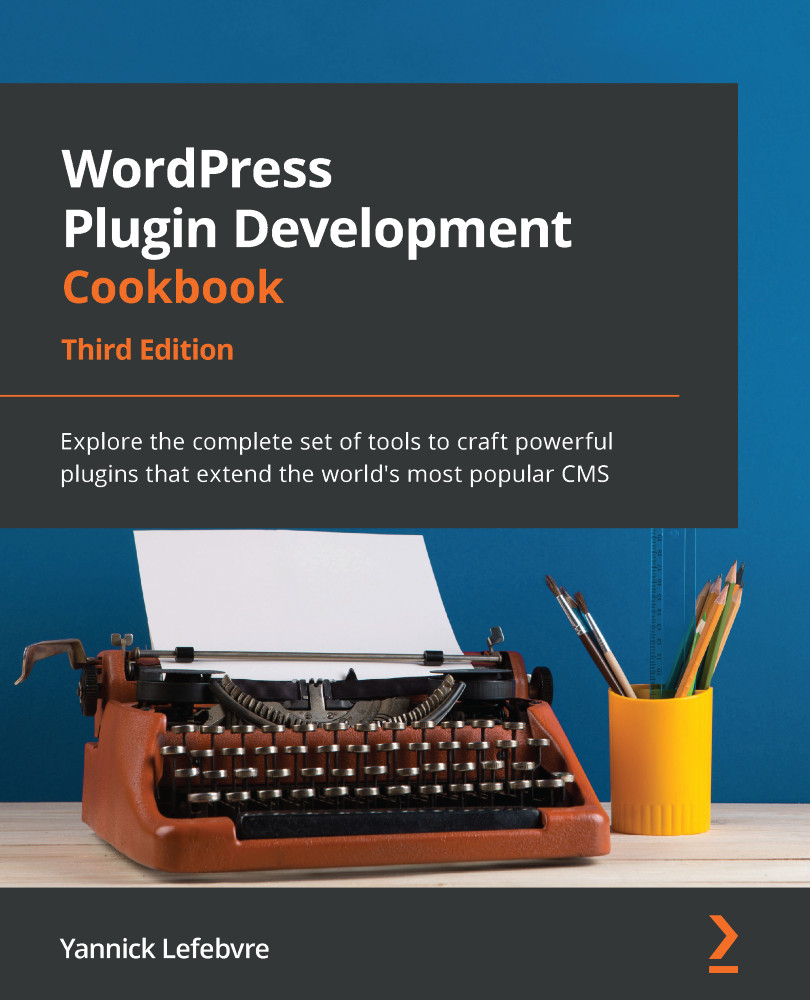
WordPress Plugin Development Cookbook
By :
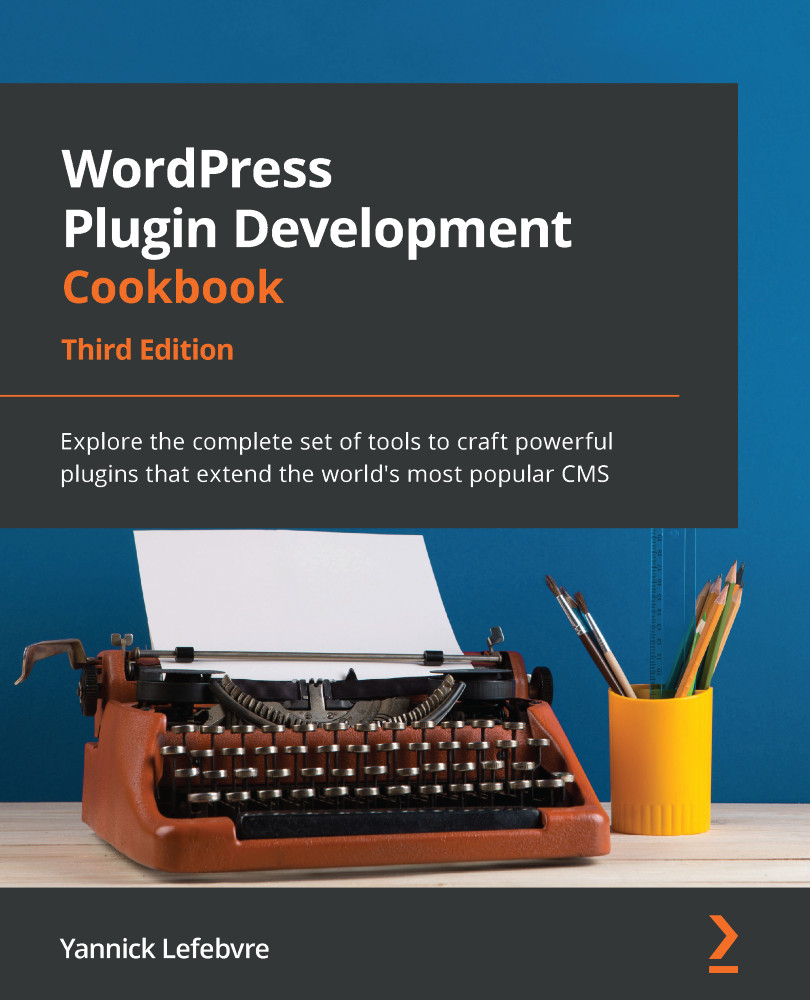
So far, all the plugin examples that have been covered in this chapter have been written using the procedural PHP programming style. In this style, all functions are declared directly in the main body of the plugin and the hook registration functions have direct access to these functions.
WordPress plugins can also be written using an object-oriented PHP approach. This recipe shows how the code from the previous recipe can be restructured to be written in object-oriented PHP.
You should have already followed the Loading a style sheet to format plugin output recipe to have a starting point for this recipe. Alternatively, you can download the resulting code (ch2/ch2-private-item-text/ch2-private-item-text-v2.php
) for that recipe from the book's GitHub page.
Follow these steps to transform an existing plugin's code into object-oriented PHP:
ch2-private-item-text
directory and rename the copy ch2-oo-private-item-text
.ch2-oo-private-item-text.php
.Chapter 2 - Object-Oriented - Private Item Text
.class CH2_OO_Private_Item_Text { function __construct() { } } $my_ch2_oo_private_item_text = new CH2_OO_Private_Item_Text();
add_shortcode
and add_action
functions to be placed inside of the class constructor method (__construct
).add_shortcode
and add_action
functions as follows:add_shortcode( 'private', array( $this, 'ch2pit_private_shortcode' ) ); add_action( 'wp_enqueue_scripts', array( $this, 'ch2pit_queue_stylesheet' ) );
ch2pit_private_shortcode
and ch2pit_queue_stylesheet
functions inside of the class body (after the __construct
method and before the class closing bracket).The code changes that we applied to the plugin first declare a class for all of our plugin's functionality and also contain a constructor method for that class. The __construct
method is called once, as soon as the class is instantiated by the last line in the plugin's code, and can be used to associate custom functions with all action hooks, filter hooks, and shortcodes.
The main benefit of using an object-oriented approach is that you don't have to be as careful when naming your hook callbacks and all other functions, since these names are local to the class and can be the same as function names declared in any other classes or in procedural PHP code.
If you enjoy object-oriented plugin development and create a lot of plugins, you might benefit from using a boilerplate generator.
By visiting the WordPress plugin boilerplate generator (https://wppb.me/), you can easily create code that needs to be written each time you create a plugin. After entering basic data about your plugin, you will receive a download with the core structure for your new plugin. This template contains a number of object-oriented concepts that are best suited to developers who are well versed in object-oriented programming.